Hi Guys!
Are you on the way to diving deep into Python programming?
Let’s have some code practice together.
Have you heard of Caesar Cipher? What is it actually?
What is Caesar’s Cipher?
Caesar Cipher is the simplest method of encoding messages.
This encryption technique is used to substitute the actual messages with a fixed number of shifts to make them encrypted.
Let’s understand by taking a simple plain text and converting it to cipher text.
Plain text: Hello
Shift: 5
Cipher text: mjqqt
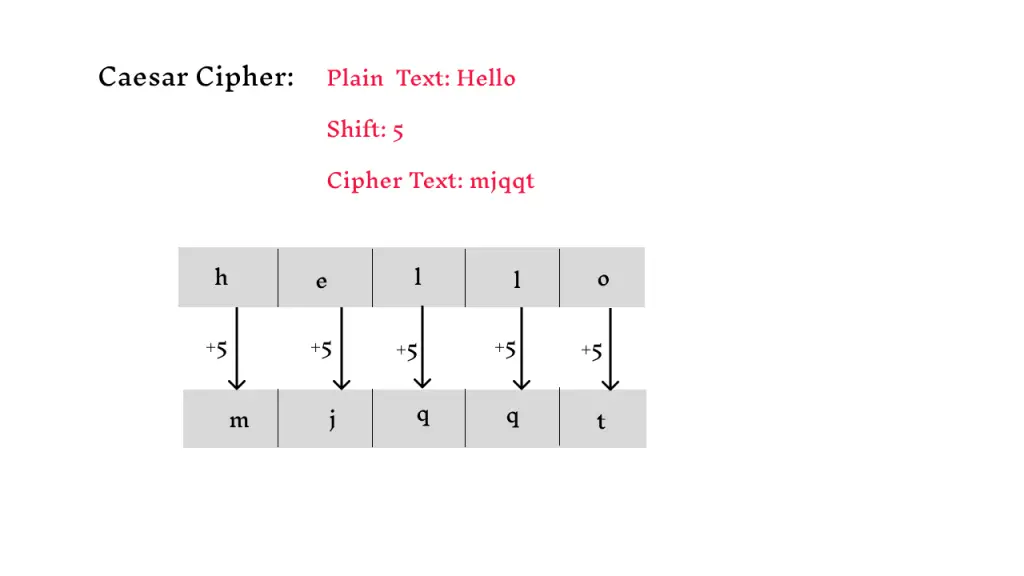
Interesting Right?
In this blog, your task is to write a code to execute the above scenario with one more twist that creates a function for both encryption and decryption so that if the user uses the cipher text “mjqqt” it can also give output as “Hello”.
Quick Info:💡
Step 1: Ask the user whether he/she needs to encode or decode.
Step 2: Enter the text message
Step 3: Ask for the shift, how much shift is required in substitution?
Step 4: Perform the required encryption or decryption and display the output.
Code for Caesar Cipher Program
Are you prepared for the challenge at hand?
Just wait a moment! First, I’d love for you to take the lead and give it a shot.
If you’ve already given it a try, that’s fantastic! If not, I’m here to assist, refine, and enhance your code. The code you’re seeking is right below.
Python Code:
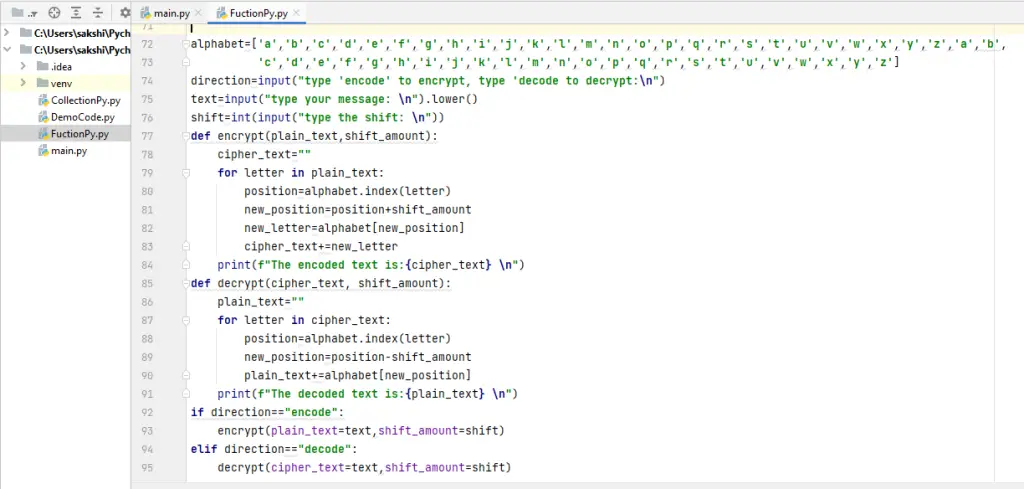
Output:
For encoding:
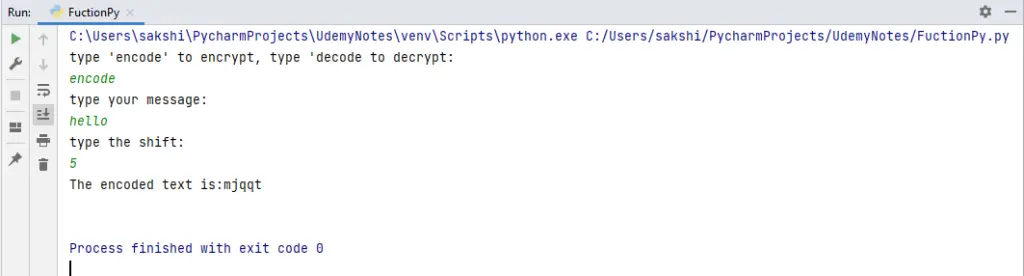
For decoding:
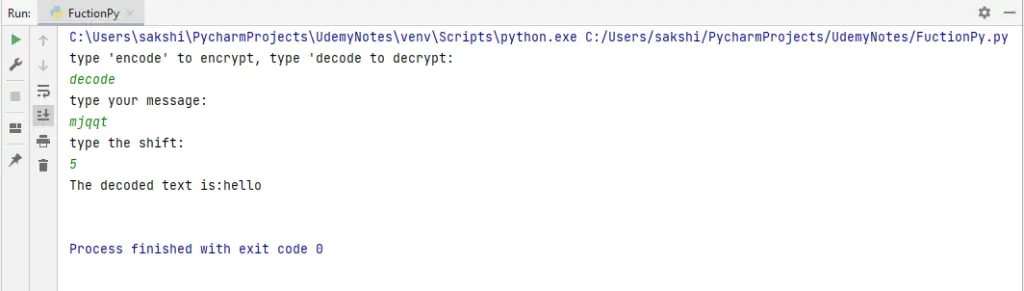
Want to check the code? Copy and paste the below code to Python IDE.
alphabet=['a','b','c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z','a','b','c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z']
direction=input("type 'encode' to encrypt, type 'decode to decrypt:\n")
text=input("type your message: \n").lower()
shift=int(input("type the shift: \n"))
def encrypt(plain_text,shift_amount):
cipher_text=""
for letter in plain_text:
position=alphabet.index(letter)
new_position=position+shift_amount
new_letter=alphabet[new_position]
cipher_text+=new_letter
print(f"The encoded text is:{cipher_text} \n")
def decrypt(cipher_text, shift_amount):
plain_text=""
for letter in cipher_text:
position=alphabet.index(letter)
new_position=position-shift_amount
plain_text+=alphabet[new_position]
print(f"The decoded text is:{plain_text} \n")
if direction=="encode":
encrypt(plain_text=text,shift_amount=shift)
elif direction=="decode":
decrypt(cipher_text=text,shift_amount=shift)
Wrapping it Up!
Congratulations to you for dedicating time and effort to coding!
Continuous practice and learning are key to becoming a better coder.
If you have any coding questions or need assistance with anything related to programming, feel free to ask. Cheers!😊
Leave a Reply