As you must be aware that we can type number, character, string, decimal, float etc in the java programming language.
But, you might be confused that “how the computer will understand which type of and size it has to print?“
For this, the language uses the Data Types concept to understand the type and size of the variables in Java.
So, let’s start Data Types in Java.
What is Data Types?
Data types specify the different type and size that can be stored in the variable.
Java has a rich implementation in the data types.
Types of Data Types
In Java, Data Types are classified into 2 parts:
- Primitive Data Types
- Non-Primitive Data Type
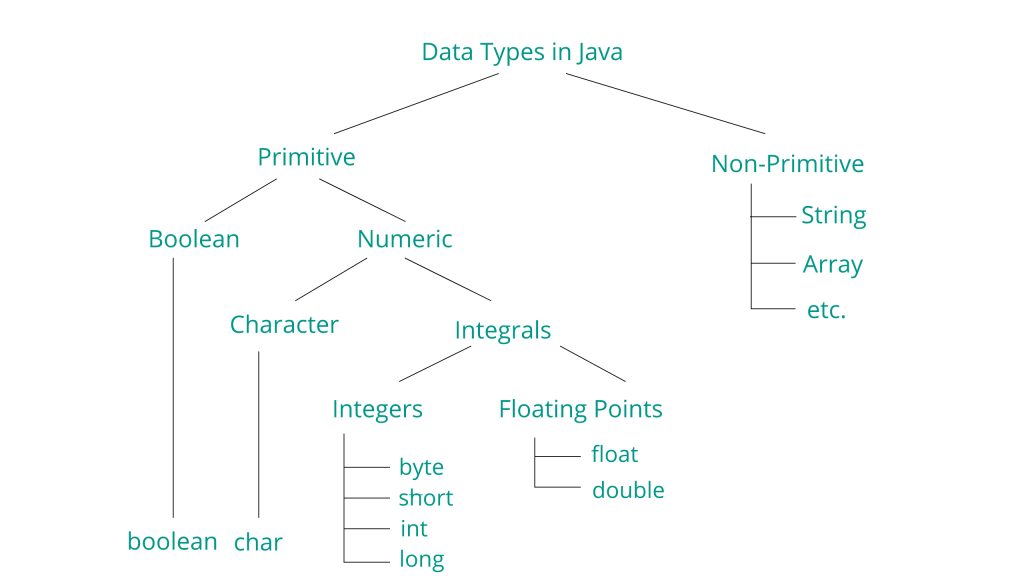
1. Primitive Data Types
Primitive Data types are pre-defined data types. It can’t be further divided into simpler type.
For example, Integer is a primitive data type, once it is declared its type cannot be changed, but its value can be changed according to users need.
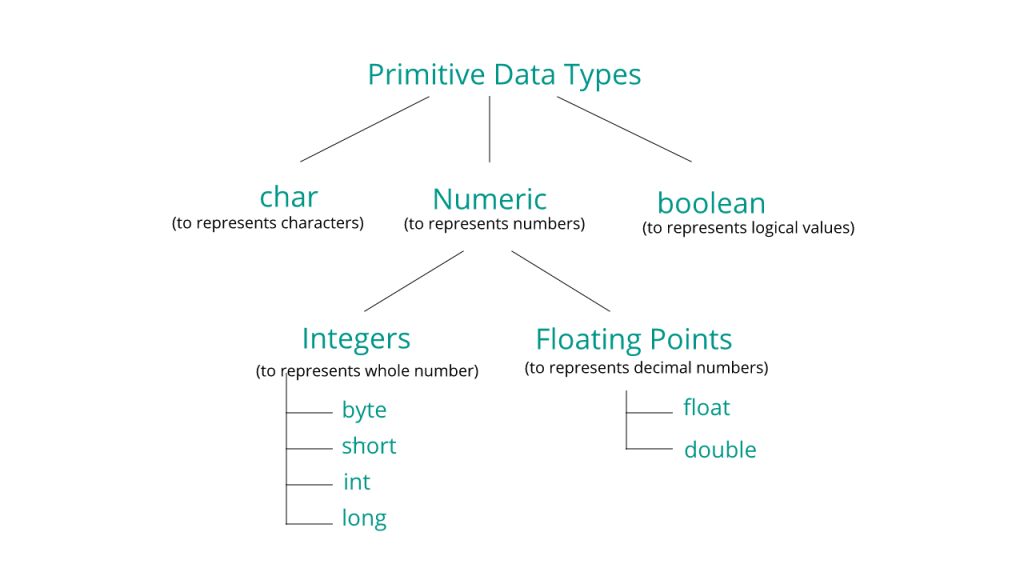
In Primitive data types, there are total 8 types:
- char data type
- byte data type
- short data type
- int data type
- long data type
- float data type
- double data type
- boolean data type
‘char’ Data Type
Default Value
Default Size
Minimum Range
Maximum Range
char
‘\u0000’
2 byte
0
65,535
Char type uses single 16-bit Unicode character ranging from ‘\u0000’ (or 0) to ‘\uffff’ (or 65,535).
Since, ‘char’ works with Unicode so it can hold alphabet characters, currency characters or other characters that come under Unicode.
Example for ‘char’:
public class CharDataType {
public static void main(String args[]){
char ch='a'; //alphabet character
System.out.println("Alphabet is: " +ch);
char ch2='$'; //currency character
System.out.println("Currency is: " +ch2);
char ch3= '&'; //other character
System.out.println("Other character: " +ch3);
}
}
//Output:
Alphabet is: a
Currency is: $
Other character: &
‘byte’ Data Type
Default Value
Default Size
Minimum Range
Maximum Range
byte
0
1 byte
-128
127
It is a 8-bit signed two’s complement integer ranging from -128 to 127.
Actually, a byte is used to save memory in the array where memory saving is most required. It takes less space compared to ‘int’ data type
Example for ‘byte’:
public class ByteDataType {
public static void main(String args[]) {
byte val = 50; //positive number
byte val2 = -112; //negative number
System.out.println("Value is: " + val);
System.out.println("Value is: " + val2);
}
}
//Output:
Value is: 50
Value is: -112
‘short’ Data Type
Default Value
Default Size
Minimum Range
Maximum Range
short
0
4 byte
-32,768
32,767
The short data type is a 16-bit signed two’s complement integer ranging from -32,768 to 32,767.
The short data type can also be used to save memory just like byte as it is 2 times smaller than an integer.
Example for ‘short’:
public class ShortDataType {
public static void main(String args[]) {
short val = 32200; //positive number
short val2 = -31700; //negative number
System.out.println("Value is: "+val);
System.out.println("Value is: "+val2);
}
}
//Output:
Value is: 32200
Value is: -31700
‘int’ Data Type
Default Value
Default Size
Minimum Range
Maximum Range
int
0
4 byte
-2,147,483,648
2,147,483,647
The int data type is a 32-bit signed two’s complement integer.
The int data type is generally used as a default data type for integral values unless if there is no issue about memory size.
A ‘int’ data type can hold upto 10 digits.
Example for ‘int’:
public class IntDataType {
public static void main(String args[]) {
int num=438;
int num2=18408;
System.out.println("Number is: "+num);
System.out.println("Number is: "+num2);
}
}
//Output:
Number is: 438
Number is: 18408
‘long’ Data Type
Default Value
Default Size
Minimum Range
Maximum Range
long
0L
8 byte
-9,223,372,036,854,775,808(-2^63)
9,223,372,036,854,775,807(2^63 -1)
The long data type is a 64-bit two’s complement integer ranging from -9,223,372,036,854,775,808(-2^63) to 9,223,372,036,854,775,807(2^63 -1)(inclusive).
The long data type is used when you need a range of values more than those provided by ‘int’ data type.
A ‘long’ data type can hold upto 19 digits.
Example for ‘long’:
public class LongDataType {
public static void main(String args[]) {
long num=4384572369827491275L; //positive number
long num2=-184088754837587345L; //negative number
System.out.println("Number is: "+num);
System.out.println("Number is: "+num2);
}
}
//Output:
Number is: 4384572369827491275
Number is: -184088754837587345
‘float’ Data Type
Default Value
Default Size
Minimum Range
Maximum Range
float
0.0f
4 byte
–
–
The float data type is a single-precision 32-bit IEEE 754 floating point whose range is unlimited.
It is recommended to use a float (instead of double) if you need to save memory in large arrays of floating-point numbers.
Example for ‘float’:
public class FloatDataType {
public static void main(String args[]) {
float num=32.5673264f;
float num2=-344.65f;
System.out.println("Number is: "+num);
System.out.println("Number is: "+num2);
}
}
//Output:
Number is: 32.567326
Number is: -344.65
‘double’ Data Type:
Default Value
Default Size
Minimum Range
Maximum Range
double
0.0 or 0.0d
8 byte
–
–
The double data type is a double-precision 64-bit IEEE 754 floating-point whose range is unlimited.
The double data type is generally used for decimal values just like float.
Example for ‘double’:
public class DoubleDataType {
public static void main(String args[]) {
double num=32.5673264;
double num2=-344.65d;
System.out.println("Number is: "+num);
System.out.println("Number is: "+num2);
}
}
//Output:
Number is: 32.5673264
Number is: -344.65
‘boolean’ Data Type
Default Value
Default Size
Minimum Range
Maximum Range
boolean
false
1 bit
–
–
The Boolean data type is used to store only two possible values: ‘true’ means 1 and ‘false’ means 0.
This data type is used for simple flags that track true/false conditions and specify only one-bit information.
Example for ‘boolean’:
public class BooleanDataType {
public static void main(String args[]) {
boolean result = true;
boolean ischeated = false;
System.out.println("result: "+result);
System.out.println(("ischeated: "+ischeated));
}
}
//Output:
result: true
ischeated: false
2. Non-Primitive Data Types
These data types are not actually defined by the programming language but are created by the programmer.
They are also called “reference variables” or “object type” since they reference a memory location which stores the data.
Some of the non-primitive data types are:
- String
- Integer
- Float
- Arrays
- HashMap etc.
Note: A primitive type starts with a lowercase letter, while non-primitive types start with an uppercase letter.
‘String’ Data Type
Strings are used for storing text.
A String contains a collection of characters surrounded by double quotes.
Example for ‘String’:
// Creating the variable type 'String' and assign it a value.
public class StringType {
public static void main(String args[]) {
String name="AspiringCoders"; //using literals
System.out.println("Name is: " +name);
}
}
//Output:
Name is: AspiringCoders
‘Array’ Data Type
Array is collection of similar data types stored in the continuous memory allocation.
We will study the array topic in detail in Array tutorials.
Example for ‘Array’:
// One-dimensional array example
public class ArrayType {
public static void main(String args[]) {
int a[]=new int[2];
a[0]=1;
a[1]=2;
System.out.println("Array at 0 position is: "+a[0]);
System.out.println("Array at 1 position is: "+a[1]);
}
}
//Output:
Array at 0 position is: 1
Array at 1 position is: 2
Note: Array starts from the 0th position known as First Index in array.
Leave a Reply