In the previous tutorial, we learnt about the ‘Hello World’ Explained.
And, now it’s time to proceed one step further on Java tutorials.
As we have seen the term variables very first in our maths book when we start learning algebra topic. Right!
But, what it actually means in Java? Is it the same here or different?
Today, we will learn about the variables in Java and also its types with code examples.
What is a Variable?
When we want to store any information, we store it in the address of the computer.
Instead of remembering the complex address where we have stored the information, we name that address. The naming of an address is called a variable.
The variable is the name of a memory location to hold data.
In other words, the variable is a name which is used to store a value of any type during program execution. To indicate the storage area each variable has given a unique name.
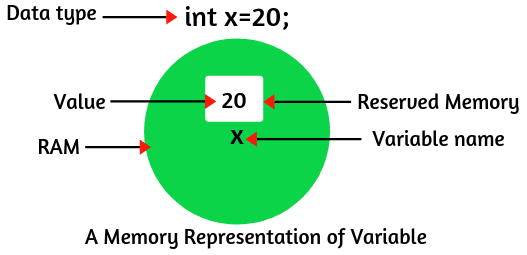
But, before we proceed further let’s have a look at Fun Fact that will help you learn variables easily.
Fun fact: There are a couple of conventions that you should know about variables:
- Variables need to be one word: they can’t have spaces, so that’s why you’ll see names like “randomly_generated_value” (using underscores) or “randomlyGeneratedValue” (camel case) instead of “randomly generated value”.
- Variables can start with letters (a-z) or(A-Z), underscore(_) or a dollar sign($).
- Some invalid variable names are:
99sheets, @sheet, !val, &char (a variable can’t start with a digit or other special symbols except for dollar)
work+nowork (contains character “+” which is not permitted)
- Names of variables should be meaningful so that you can be reminded of what information it contains.
Ways to Write Variables
After understanding the meaning of variables, you must be thinking that
How can we declare(create) and assign values to the variables?
It can be done in two ways:
1. Declaring a Variable
For declaring a variable, you must specify its data type, leave at least one space then name that variable and end it with a semicolon(;).
Syntax for Variable Declaration:
int count; // variable declaration
Here, int is a data type for an integer number
the count is a variable
; (semicolon) is for ending a line.
Note: We can assign value later according to needs.
2. Declaring and Initializing a Value
Another way is initializing the value of the variable’s value simultaneously while declaring it.
Syntax for Declaring and Initializing a Variable :
int count=40; // variable declaration and initialization
Here, int is a data type for an integer number
count is a variable
40 is the value assigned to the variable count.
Types of Variables
There are three types of variables in Java:
- Local variable
- Instance variable
- Static variable
Local Variable
A local variable is a variable that is declared within the body of a method. And, you can use it only within that method.
A local variable can be declared in methods, constructors or blocks.
Other methods in the class aren’t even aware that the variable exists. i.e. Local variables are created when the method is entered and the variable gets destroyed once it exits the method.
There is no default value for the local variable so the local variable should be declared and an initial value should be assigned before the first use.
Syntax for Local Variable:
class{
constructor{
// use local variable within constructor
}
method{
// use local variables within method
block{
//use local variables within block
}
}
}
Examples of Local Variable:
// Sample 1: Local Variable
public class LocalVariable {
public static void main(String []args){
int a=10;
System.out.println("Value of a:" +a); //accessible
{
int b=20; //Local Variable
System.out.println("Value of b:" +b); //accessible
}
System.out.println("Value of a:" +a); // accessible
}
}
// Output:
Value of a:10
Value of b:20
Value of a:10
// Sample 2: Local Variable Scope (Warning!)
public class LocalVariable {
public static void main(String []args){
int a=10;
System.out.println("Value of a:" +a); // can be accessed
{
int b=20; //Local variable
System.out.println("Value of a:" +a); // can be accessed
System.out.println("Value of b:" +b); //can be accessed (local)
}
System.out.println("Value of b:" +b); // it will show an error
System.out.println("Value of a:" +a); // can be accessed
}
}
WARNING! This second last line System.out.println(“Value of b:” +b); will show an error and lead to a compilation error. Why did it happen?
According to local variable syntax, it can only be accessible within the specific blocks {…..} i.e. “area between opening and closing curly brace” defines a scope of a variable.
And, outside that block, the variable is not known to other methods of the class.
Instance Variable
An Instance variable is declared inside the class but outside any block, method or constructor.
When space is allocated for an object in the heap a slot for each instance value is created.
An instance variable is created when the object is created with the use of the “new” keyword and destroyed when the object is destroyed.
An Instance variable is visible to all the methods or blocks in the class.
Example for Instance Variable:
/* Instance variables can be declared in the body of the class before or after use */
public class InstanceVariable {
public void test()
{
int b=10; //Local Variable
System.out.println("Value of b: " +b);
System.out.println("Value of a: " +a);
}
int a=50; //Instance Variable
public static void main(String []args) {
InstanceVariable iv=new InstanceVariable();
iv.test();
}
}
// Output:
Value of b: 10
Value of a: 50
/* Instance variables can be assigned during the declaration or within the constructor */
public class InstanceVariable2 {
float a = 10.5f; //Instance variable
int myVar;
InstanceVariable2() // default constructor
{
myVar = 20;
}
public void test() {
boolean b = true; // Local Variable
System.out.println("Value of a: "+a);
System.out.println("Value of a: "+b);
System.out.println("Value of a: "+myVar);
}
public static void main(String []args){
InstanceVariable2 iv2=new InstanceVariable2();
iv2.test();
}
}
// Output:
Value of a: 10.5
Value of b: true
Value of myVar: 20
Note: 1. Access modifier can be given for the Instance Variable.
2. Instance variables have a default value. ‘0’ for the Integer, ‘False’ for the Boolean and ‘Null’ for the Object References.
Static Variable
Static variables are declared with the ‘static’ keyword in the class but outside the methods, blocks or constructors.
We can say that – Static variables are the variables in the class which are initialized only once in the program, at the start of the execution.
There would be only one copy of each class variable per class, regardless of how many objects are created from it.
Static variables are normally used for the constants.
Syntax for Static Variables:
static data_type variable_name;
For syntax, first a ‘static’ keyword, then datatype for variable and last variable_name.
Examples of Static Variable:
//Sample 1: Default value for Static Variables
public class StaticVariable {
static int intVar;
static float floatVar;
static Object obj;
static boolean boolVar;
public static void main(String agrs[]){
System.out.println("Default value of intVar: " +intVar);
System.out.println("Default value of floatVar: " +floatVar);
System.out.println("Default value of obj: " +obj);
System.out.println("Default value of boolVar: " +boolVar);
}
}
//Output:
Default value of intVar: 0
Default value of floatVar: 0.0
Default value of obj: null
Default value of boolVar: false
// Sample 2: static vs non-static
public class StaticVariable2 {
public static int Scounter=0;
//Static variable
public int counter=0;
public StaticVariable2() {
Scounter++;
counter++;
}
public static void main(String []args){
StaticVariable2 t=new StaticVariable2();
StaticVariable2 t1=new StaticVariable2();
StaticVariable2 t2=new StaticVariable2();
System.out.println("Final value of Counter:" + t2.counter);
System.out.println("Final value of SCounter:" + t2.Scounter);
//Static Variable
}
}
// Output:
Final value of Counter: 1
//non-static variable output
Final value of SCounter: 3
//Static variable output
Explanation of the Above Code:
For counter variable; Initially, counter=0;
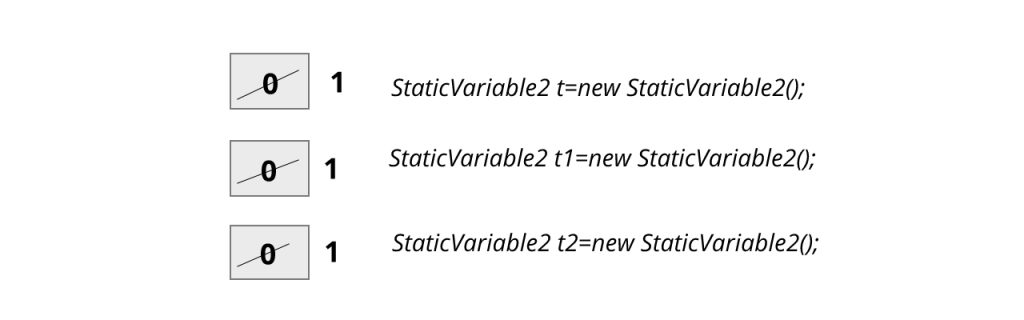
For Scounter variable; Initially, Scounter=0;
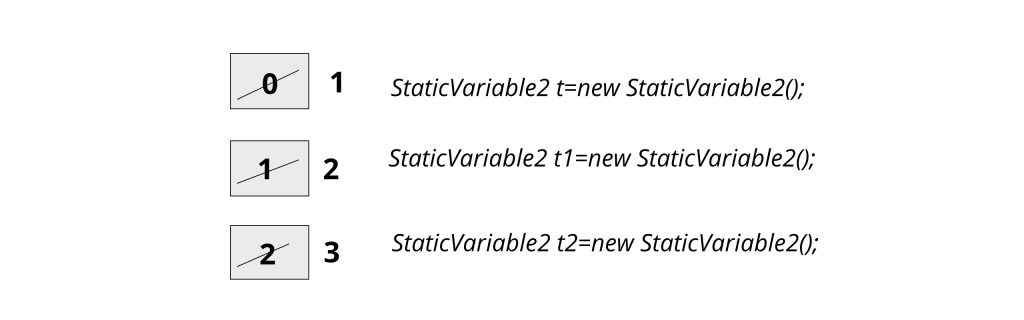
Leave a Reply