We have seen how to compile and run the Java code using different methods in the previous page i.e. My First Java Program. But, what does code actually mean?
What do several lines say? Why couldn’t we write Java code in one line like Python?
To get the answers to these basic questions first, we have to understand the different keywords used in the ‘Hello World’ program.
If we create a simple Java ‘Hello World’ program to print ‘Hello World’ in the output console, it will be like this.
// Hello World Program
public class HelloWorldProgram
{
public static void main(String args[])
{
System.out.println("Hello World");
}
}
And one thing, the filename and Java class name should be the same. Visit this page to understand: Why the filename in Java should be same as the class name?
Parameters used in the ‘Hello World’ Program
public: It is an access specifier. Public means this function can be accessed from anywhere in the whole program.
class: class keyword is used to declare classes in Java. In Java, every code is written inside a class.
static: static is again a keyword used to make a function static. To execute a static function you do not have to create an Object of the class. The main() method here is called by JVM, without creating any object for the class.
void: It is the return type, which means this function will not return anything.
main: main() method is the most important method in a Java program. This is the method from where the Java compiler starts its execution, hence all the logic must be inside the main() method. If a Java class is not having a main() method, it causes a compilation error.
String[] args: This represents an array whose type is String and name is args. We will discuss more about arrays in the section on Java Array.
System.out.println: This is used to print anything on the console like cout in C++ or printf in C language.
1. // Hello World Program
In Java, anything that starts with // is considered a comment. Comments are statements or codes which are not executed by the compiler.
Generally, comments make the coding more understandable and readable to the user by providing information about any line of code.
And, comments are also used to hide some lines of code for a specific time.
Comments are of two types: Single-line comments(//) and Multi-line comments(/*……………*/).
2. public class HelloWorldProgram
{...........}
As Java is an object-oriented programming language so every program begins with the class definition in Java.
Here, ‘HelloWorldProgram’ is the name of the class and the public is an access specifier.
Public means that the class is visible to all other classes and accessed by other classes.
3. public static void main(String args[])
{.........}
In Java, the main method is the entry point for any program.
The Main has to be public so that when JVM invokes it, program execution gets started.
The void return type is used here because JVM doesn’t require any value from the main method.
There must be a question in your mind: Can we change the order to static public void main?
Yes! we can change the order of statements like this. The compiler will not show any error during compilation or runtime.
In Java, we can declare access specifier in any order with the condition that the main method should be at last and void should come second last.
Now the question arises, Can there be more ways to write String[] args?
Yes! There are different ways to write this statement also because as we discussed earlier it stores Java command-line arguments and is an array of type java.lang.String class.
Ways to write this statement: String[] args, String []args, String… args
4. System.out.println("Hello World");
This statement is used to print an argument that is passed to it i.e. it is used to print the output on the output console.
Here, System is a class, out is a variable, println() is a method and “Hello World” is a parameter passed to the println() method.
Actually, there are two methods to print the statement
- print(): This method is used to simply print the text on the output console, it doesn’t add a new line after execution.
- println(): This method is used to add the new line after printing text on the output console.
To access the deep knowledge on this statement, click here.
Life cycle of Java program execution
The life cycle of a Java program tells us right from the point when you type the source code in the text editor to the time it shows the output.
After understanding the different parameters used in printing the ‘Hello World”, You must be excited to know “How actually the internal process takes place?” Right!
And, to get through the answer you have to understand the diagram provided below:
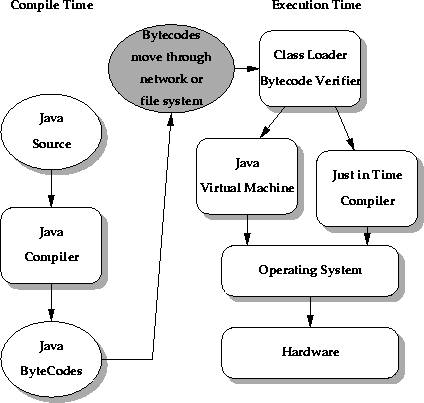
Let us assume, we have source code AspiringCoders.java with .java extension and our target is to run this source code on the hardware.
First, the source code gets through the compilation phase where the source code with .java extension gets converted into the bytecode with .class extension.
At this, the high-level language gets converted to machine-level language which is also known as bytecode.
This bytecode can run on any platform and only takes a JVM for a specific OS. It internally invokes the classloader for loading the .class file from the hard disk to JVM’s address space.
A bytecode verifier is used to verify the code to avoid runtime failures. After verification, Bytecode is executed with the help of OS. Thus, the whole program is get executed by the OS and JVM.
Leave a Reply