In this tutorial, we will learn about the comparison in C++ i.e. find the largest among three numbers using C++.
Quick Info:💡
↪ Largest among three numbers means a program through which computer will able to provide you with the largest number among three numbers entered by the user.
↪ For example, suppose user will enter the three numbers- 23, 45, 67 then, after the execution of code you’ll get the result as 67, which is largest among given three numbers.
↪ Here’s the following steps to to find the largest among three:
- First, consider any three numbers
- Second, apply the conditions to find the result
- Third, print the result on the output console
↪ Here’s the ways to perform the above task:
- If statement
- If-else statement
- Else if statement
- Ternary operator
Find the Largest Among Three Numbers Using If Statement
Here’s a simple method to find the largest among three numbers using if statements.
If statement is a conditional statement which gets executed if the given condition is evaluated true.
Example:
#include<iostream.h>
#include<conio.h>
void main()
{
int first_number, second_number, third_number;
cout<<"Enter integer for first number:";
cin>>first_number;
cout<<"Enter integer for second number:";
cin>>second_number;
cout<<"Enter integer for third number:";
cin>>third_number;
if(first_number>second_number&&first_number>third_number)
cout<<"First number is largest";
if(second_number>first_number&&second_number>third_number)
cout<<"Second number is largest";
if(third_number>first_number&&third_number>second_number)
cout<<"Third number is largest";
getch();
}
//Output:
Enter integer for first number:34
Enter integer for second number:78
Enter integer for third number:45
Second number is largest
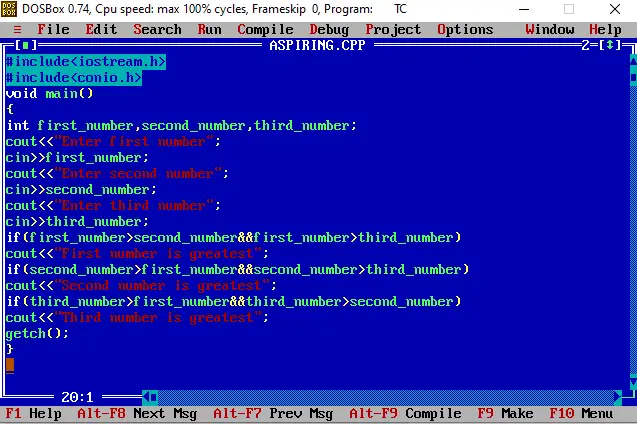
Working:
Flow of a program:
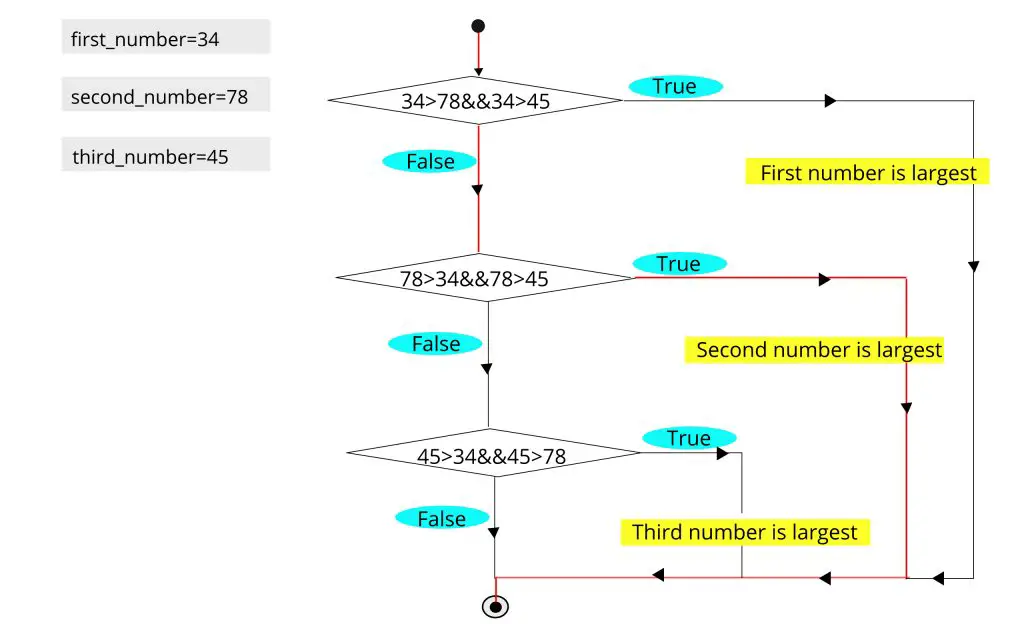
Find the Largest Among Three Numbers Using If-Else Statement
If-else statement is a another way to find the largest number among three numbers.
An if statement is followed by a optional else statement, which gets executed if the given condition is evaluated to be false.
Example:
#include<iostream.h>
#include<conio.h>
void main()
{
int first_number, second_number, third_number;
cout<<"Enter integer for first number:";
cin>>first_number;
cout<<"Enter integer for second number:";
cin>>second_number;
cout<<"Enter integer for third number:";
cin>>third_number;
if(first_number>second_number&&first_number>third_number)
cout<<"First number is largest";
if(second_number>first_number&&second_number>third_number)
cout<<"Second number is largest";
else
cout<<"Third number is largest";
getch();
}
//Output:
Enter integer for first number:34
Enter integer for second number:78
Enter integer for third number:45
Second number is largest
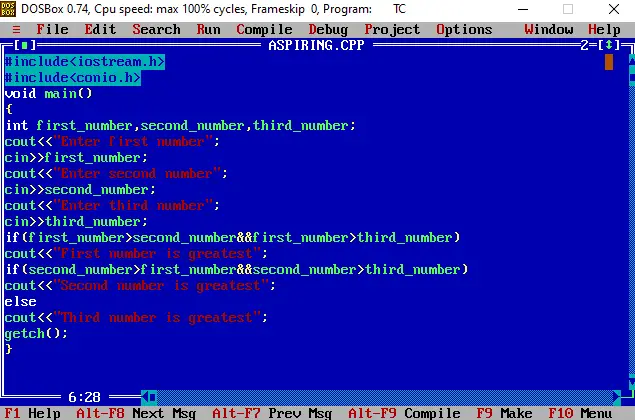
Working:
Flow of a program:
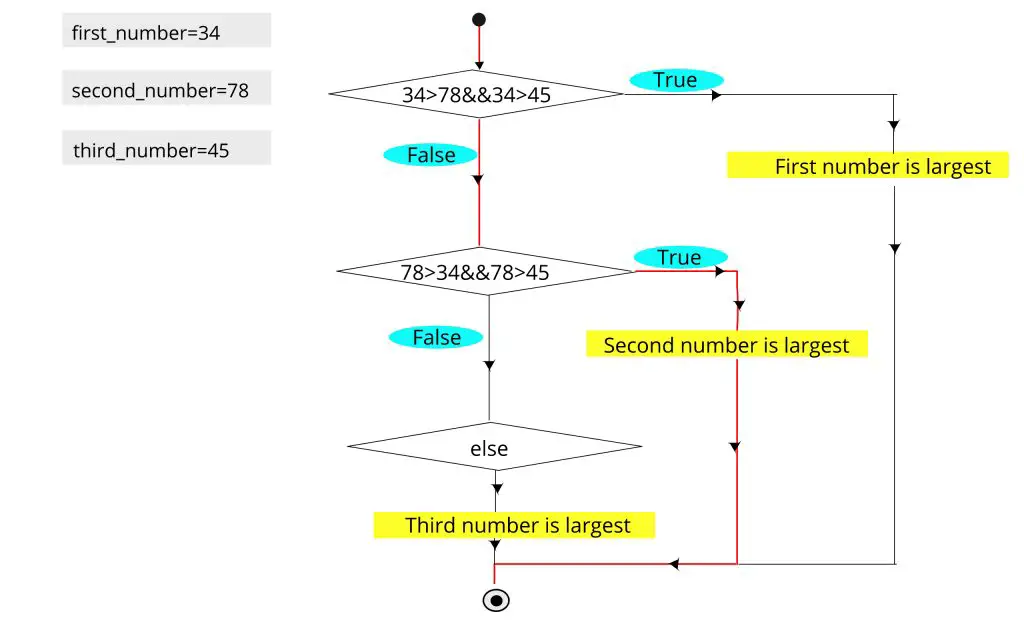
Find the Largest Among Three Numbers Using Else If Statement
Else if statement is also a easy way with little changes in if-else conditions.
Else if statement is used in a program when there is multiple decisions in if statement.
Example:
#include<iostream.h>
#include<conio.h>
void main()
{
int first_number, second_number, third_number;
cout<<"Enter integer for first number:";
cin>>first_number;
cout<<"Enter integer for second number:";
cin>>second_number;
cout<<"Enter integer for third number:";
cin>>third_number;
if(first_number>second_number&&first_number>third_number)
cout<<"First number is largest";
else if(second_number>first_number&&second_number>third_number)
cout<<"Second number is largest";
else if(third_number>first_number&&third_number>second_number)
cout<<"Third number is largest";
getch();
}
//Output:
Enter integer for first number:34
Enter integer for second number:19
Enter integer for third number:45
Third number is largest
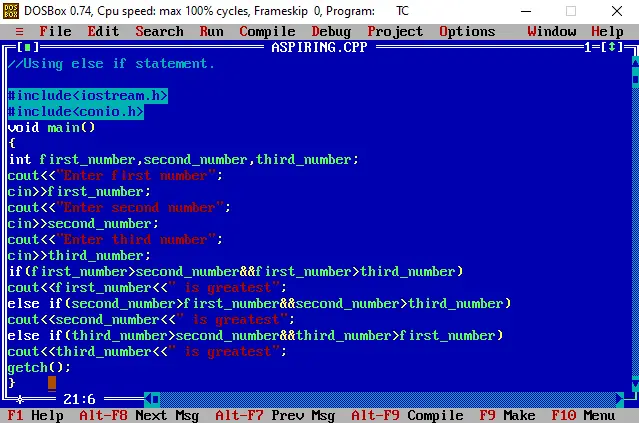
Working:
Flow of a program:
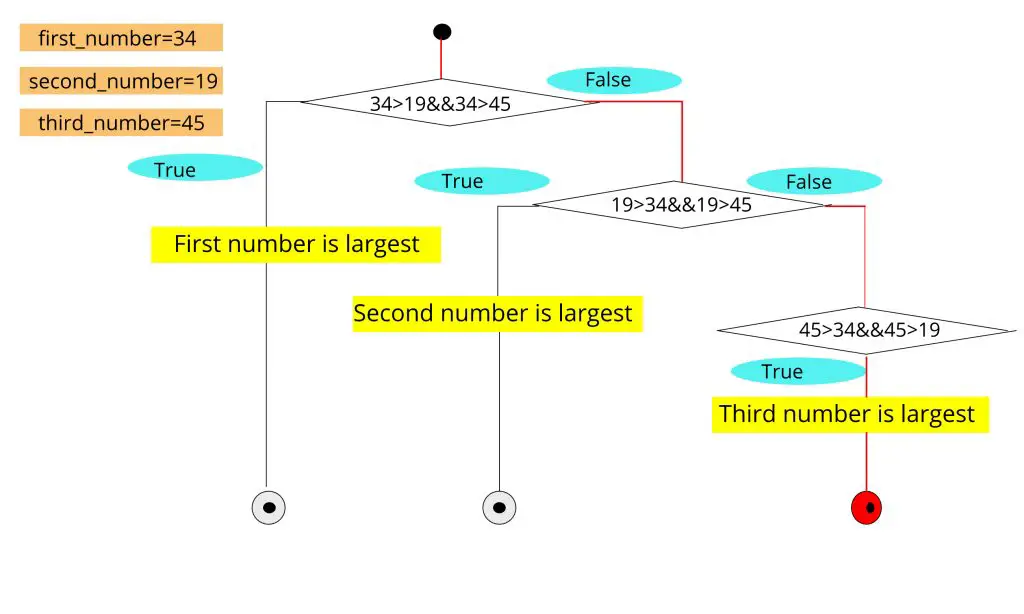
Find the Largest Among Three Numbers Using Ternary Operator
Ternary operators are those operators that work as an alternative of the if-else statement.
They are called ternary because it works on three operators (?:)
Here, the condition is evaluated and,
- If condition is evaluated to be true, first statement gets executed
- If condition is evaluated to be false, second statement gets executed
Example:
#include<iostream.h>
#include<conio.h>
void main()
{
int first_number, second_number, third_number, result, result1;
cout<<"Enter integer for first number:";
cin>>first_number;
cout<<"Enter integer for second number:";
cin>>second_number;
cout<<"Enter integer for third number:";
cin>>third_number;
result=(first_number>second_number)?first_number:second_number;
result1=(result>third_number)?result:third_number;
cout<<result1<<"is greatest";
getch();
}
//Output:
Enter integer for first number:34
Enter integer for second number:78
Enter integer for third number:45
78 is greatest
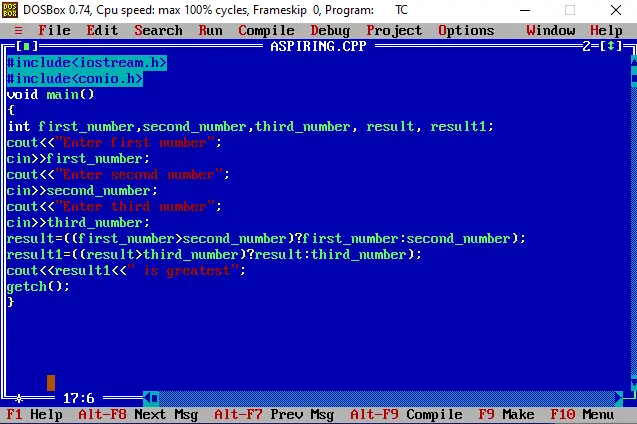
From the above examples, we understood that there are several ways to write a single program using different ways.
Sounds Interesting! Right?
Well, I hope it really helped you to know the C++ better.
And, if you liked this stay with us to get more such exciting codes to explore more in C++.
View related posts:
- C++ Program to Add Two Matrices (Multi-dimensional Array)
- C++ Program to Add Two Numbers
- C++ Program to Calculate the Simple Interest
- C++ Program to Check Armstrong Number
- C++ Program to Check Palindrome Number
- C++ Program to Check Whether a Character is Vowel or Consonant
- C++ Program to Demonstrate the Arithmetic Operators
- C++ Program to Demonstrate the Assignment Operator
- C++ Program to Display Multi-Dimensional Array
- C++ Program to Display Single Dimensional Array
- C++ Program to Find Factorial of a Number
- C++ Program to Find Out Product of Digits
- C++ Program to Find Out Sum of Digits
- C++ Program to Find Out the Area of Square
- C++ Program to Find the Fibonacci Sequence
- C++ Program to Find the Largest Among Three Numbers
- C++ Program to Reverse a Number
- C++ Program to Swap Two Numbers
- C++ Program to Transpose the Matrix (2-D Array)
Leave a Reply