In this tutorial, we will learn about the single-dimensional array in C++ i.e. how to input and print the one-dimensional array using C++.
But, before that, it’s important to know what actually array is. and what is its syntax?
Quick Info:💡
↪ An array is a collection of values of similar data types arranged at contiguous memory locations and can be accessed using indices.
↪ Array is of two types:
- Single-dimensional array
- Multidimensional array
↪ Points to be remembered: In an array, indexing always starts from zero(0).
Program to Display Single-Dimensional Array
Syntax:
datatype nameofarray[size];
- datatype– It is a data type of the value that is stored in the array.
- nameofarray– It is the name of the array.
- size– It is the number of elements that can be stored in an array.
Example:
#include<iostream.h>
#include<conio.h>
void main()
{
int i, arr[4];
cout<<"Enter the elements of array below:"<<endl;
for(i=0;i<=4;i++)
{
cout<<"Enter the element of arr["<<i<<"]:";
cin>>arr[i];
}
for(i=0;i<=4;i++)
{
cout<<"Element in arr["<<i<<"] is:"<<arr[i]<<endl;;
}
getch();
}
//Output:
Enter the elements of array below:
Enter the element of arr[0]:23
Enter the element of arr[1]:45
Enter the element of arr[2]:76
Enter the element of arr[3]:90
Enter the element of arr[4]:70
Element in arr[0] is:23
Element in arr[1] is:45
Element in arr[2] is:76
Element in arr[3] is:90
Element in arr[4] is:70
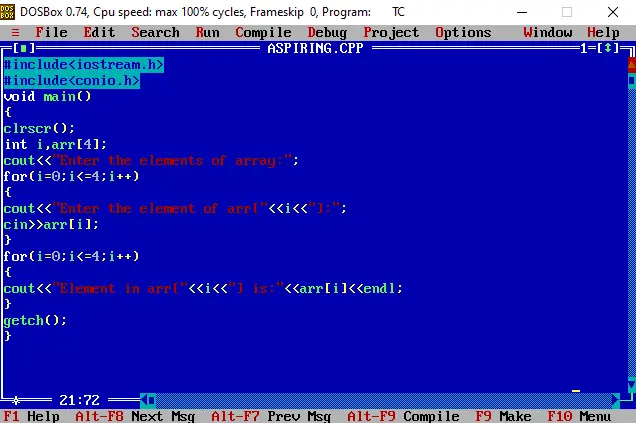
Working:
The flow of a program:
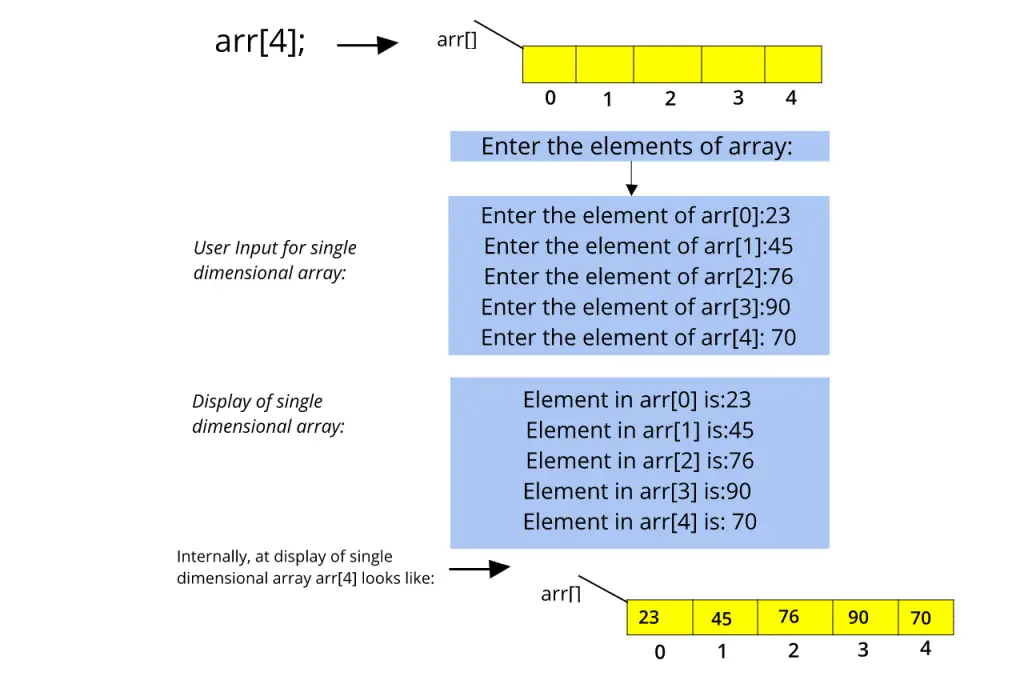
Wrapping Up:
Well, I hope it really helped you to know C++ better.
Attention reader⚠ Don’t stop learning now.
Like a single dimensional array, a multidimensional array is also an important part of the array.
Just stay with us to get more such exciting codes to explore more in C++.
View related posts:
- C++ Program to Add Two Matrices (Multi-dimensional Array)
- C++ Program to Add Two Numbers
- C++ Program to Calculate the Simple Interest
- C++ Program to Check Armstrong Number
- C++ Program to Check Palindrome Number
- C++ Program to Check Whether a Character is Vowel or Consonant
- C++ Program to Demonstrate the Arithmetic Operators
- C++ Program to Demonstrate the Assignment Operator
- C++ Program to Display Multi-Dimensional Array
- C++ Program to Display Single Dimensional Array
- C++ Program to Find Factorial of a Number
- C++ Program to Find Out Product of Digits
- C++ Program to Find Out Sum of Digits
- C++ Program to Find Out the Area of Square
- C++ Program to Find the Fibonacci Sequence
- C++ Program to Find the Largest Among Three Numbers
- C++ Program to Reverse a Number
- C++ Program to Swap Two Numbers
- C++ Program to Transpose the Matrix (2-D Array)
Leave a Reply