In this tutorial, we will learn about addition in C++ i.e. how to write a program to add two numbers using C++.
And, to know the basics of C++ and about the installation of Turbo C++, visit the C++: an overview.
Quick Info:đź’ˇ
↪Addition of two numbers is an arithmetic operation that can carry out using operators and operands.
↪We will find the sum of the two numbers and display the result as output in the output console.
Addition can be performed in different ways using C++:
- Simple method
- By user input
- By using Class
- By using Function
- Using array
Note: For the sake of simplicity, I’d only used the “int” as the data type but I recommend you try this program using different data types.
Program to Add Two Numbers Using Simple Method
Here’s a simple method that demonstrates the addition of two numbers.
Example:
#include<iostream.h>
#include<conio.h>
int main(){
int first_number=12, second_number=34, result;
result=first_number+second_number;
cout<<"ADDITION IS:="<<result;
getch();
return 0;
}
//output:
ADDITION IS:=46
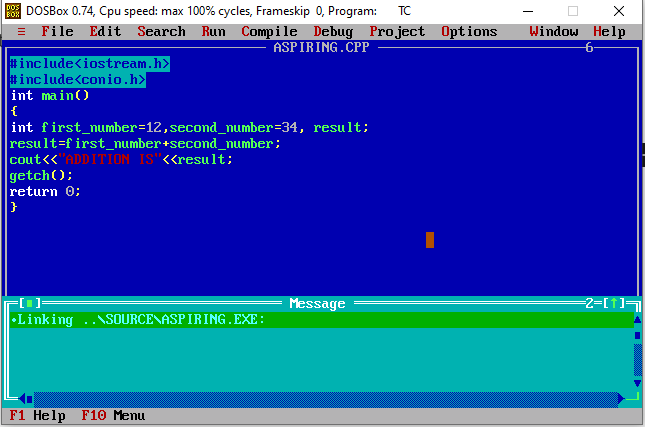
Program to Add Two Numbers Using User Input
Here, the user will be asked to enter the two integers and the sum of these two integers will be displayed as the output.
Example:
#include<iostream.h>
#include<conio.h>
int main(){
int first_number, second_number, result;
cout<<"enter integer for first_number:";
cin>>first_number;
cout<<"enter integer for second_number:";
cin>>second_number;
result=first_number+second_number;
cout<<"ADDITION IS:="<<result;
getch();
return 0;
}
//Output:
enter integer for first_number:12
enter integer for second_number:13
ADDITION IS:=25
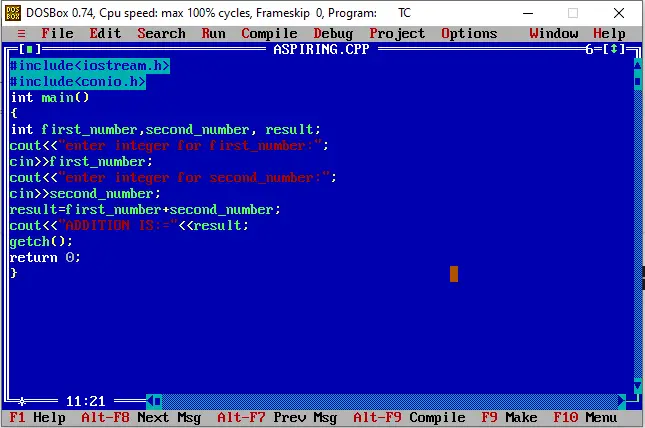
Program to Add Two Numbers Using Class
A class is a user-defined data type which makes C++ an object-oriented language.
We create a class with two functions input and display_add. Function input is used to get two integers from a user, and function display_add performs the addition and displays the result.
#include<iostream.h>
#include<conio.h>
class addition{
public:
int first_number,second_number,result;
void input(){
cout<<"enter integer for first_number:";
cin>>first_number;
cout<<"enter integer for second_number:";
cin>>second_number;
}
void display_add(){
result=first_number+second_number;
cout<<"ADDITION IS:="<<result;
}
};
void main(){
addition obj;
obj.input();
obj.display_add();
getch();
}
//Output:
enter integer for first_number:12
enter integer for second_number:13
ADDITION IS:=25
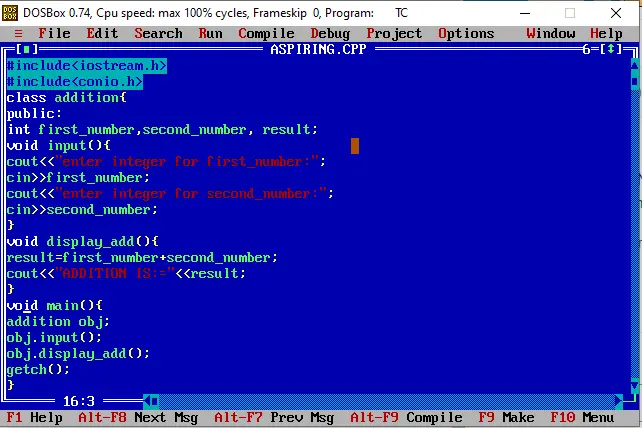
Program to Add Two Numbers Using Function
A function is a group of statements which perform a specific task.
Actually, it is a self-contained block of statements which performs its task when get executed.
For addition first, create a function add and pass the arguments for two integers.
Syntax:
return_type function_name(list of arguments)
{......}
Example:
#include<iostream.h>
#include<conio.h>
int add(int first_number, int second_number){
return first_number+second_number;
}
int main(){
int first_no=23, second_no=12;
int sum;
sum=add(first_no,second_no);
cout<<"Addition is:="<<sum;
getch();
return 0;
}
//Output:
Addition is:=35
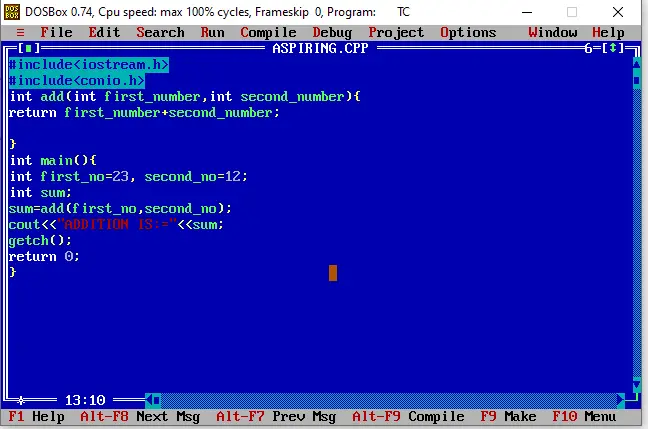
Program to Add Two Numbers Using Array
An array is a collection of similar data types stored in the contiguous memory allocation.
To perform the addition using an array, we have to first initialize the elements of arrays to store the value of two integers within it.
And, then add the elements of an array to get the value of sum as the output in the output console.
Example:
#include<iostream.h>
#include<conio.h>
void main(){
int arr[3];
arr[1];
arr[2]=12;
arr[3]=10;
arr[1]=arr[2]+arr[3];
cout<<"ADDITION IS:="<<arr[1];
getch();
}
//Output:
ADDITION IS:=22
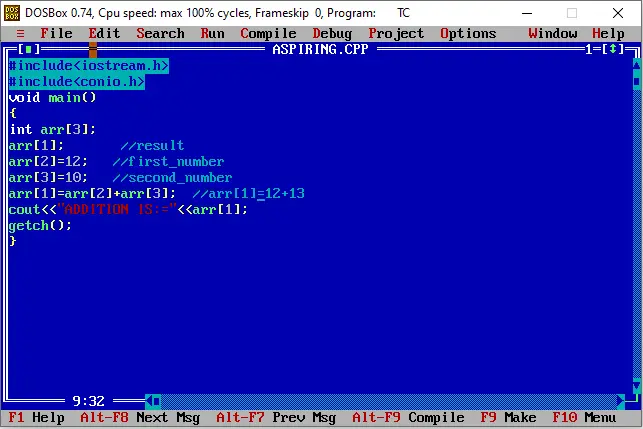
From the above examples, we understood that there are several ways to write a single program using different ways.
Sounds Interesting! Right?
Well, I hope it really helped you to know C++ better.
And, if you liked this stay with us to get more such exciting codes to explore more in C++.
View related posts:
- C++ Program to Add Two Matrices (Multi-dimensional Array)
- C++ Program to Add Two Numbers
- C++ Program to Calculate the Simple Interest
- C++ Program to Check Armstrong Number
- C++ Program to Check Palindrome Number
- C++ Program to Check Whether a Character is Vowel or Consonant
- C++ Program to Demonstrate the Arithmetic Operators
- C++ Program to Demonstrate the Assignment Operator
- C++ Program to Display Multi-Dimensional Array
- C++ Program to Display Single Dimensional Array
- C++ Program to Find Factorial of a Number
- C++ Program to Find Out Product of Digits
- C++ Program to Find Out Sum of Digits
- C++ Program to Find Out the Area of Square
- C++ Program to Find the Fibonacci Sequence
- C++ Program to Find the Largest Among Three Numbers
- C++ Program to Reverse a Number
- C++ Program to Swap Two Numbers
- C++ Program to Transpose the Matrix (2-D Array)
Leave a Reply