In this tutorial, we will learn about the addition of matrices. i.e. a program to add two matrices using a multi-dimensional array in C++.
Quick Info:💡
↪ As we know, what is a multidimensional array and how to display multidimensional array (matrices) in C++?
↪ Here, we’ll try to find out the sum of matrices using C++ programming language.
↪ Steps to perform above task:
- First, input the two identity matrices.
- Second, perform the addition of two matrices i.e. C[][]=A[][]+B[][].
- Third, display the result on the output screen.
Program to Add Two Matrices (Multi-dimensional Array)
Here’s a direct method to add two matrices in multidimensional array.
Simply, the user will be asked to enter two identity matrices then, the sum is computed and displayed to the user.
Example:
#include<iostream.h>
#include<conio.h>
void main()
{
int i,j,A[2][2],B[2][2],C[2][2];
cout<<"Enter the elements of array:"<<endl;
for(i=0;i<2;i++){
for(j=0;j<2;j++){
cout<<"Enter the elements of A["<<i<<"]["<<j<<"]:";
cin>>A[i][j];
}}
cout<<"Enter the elements of array:"<<endl;
for(i=0;i<2;i++){
for(j=0;j<2;j++){
cout<<"Enter the elements of B["<<i<<"]["<<j<<"]:";
cin>>B[i][j];
}}
cout<<"Matrix A[][] is:"<<endl;
for(i=0;i<2;i++){
for(j=0;j<2;j++){
cout<<A[i][j]<<"\t";
}
cout<<"\n";
}
cout<<"Matrix B[][] is:"<<endl;
for(i=0;i<2;i++){
for(j=0;j<2;j++){
cout<<B[i][j]<<"\t";
}
cout<<"\n";
}
cout<<"Sum of matrices is:"<<endl;
for(i=0;i<2;i++){
for(j=0;j<2;j++){
C[i][j]=A[i][j]+B[i][j];
cout<<C[i][j]<<"\t";
}
cout<<"\n";
}
getch();
}
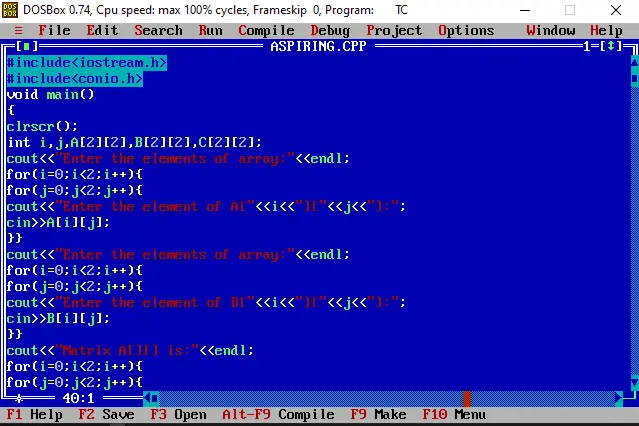
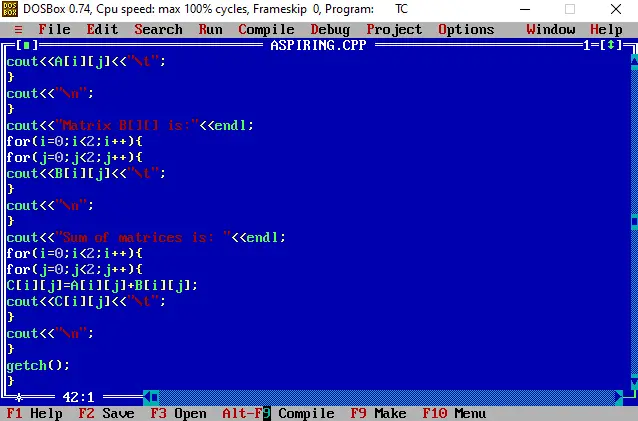
Output:
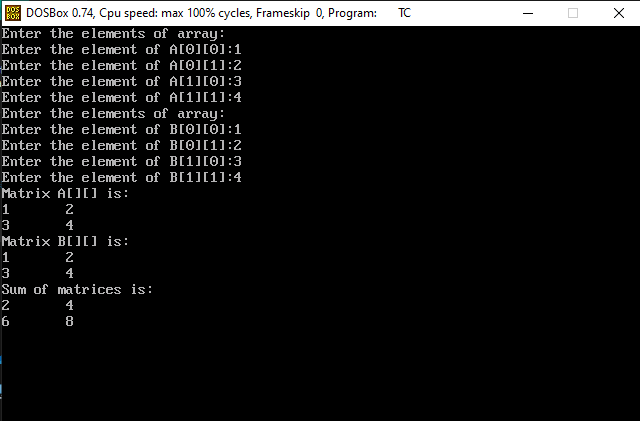
Working:
Flow of a program:
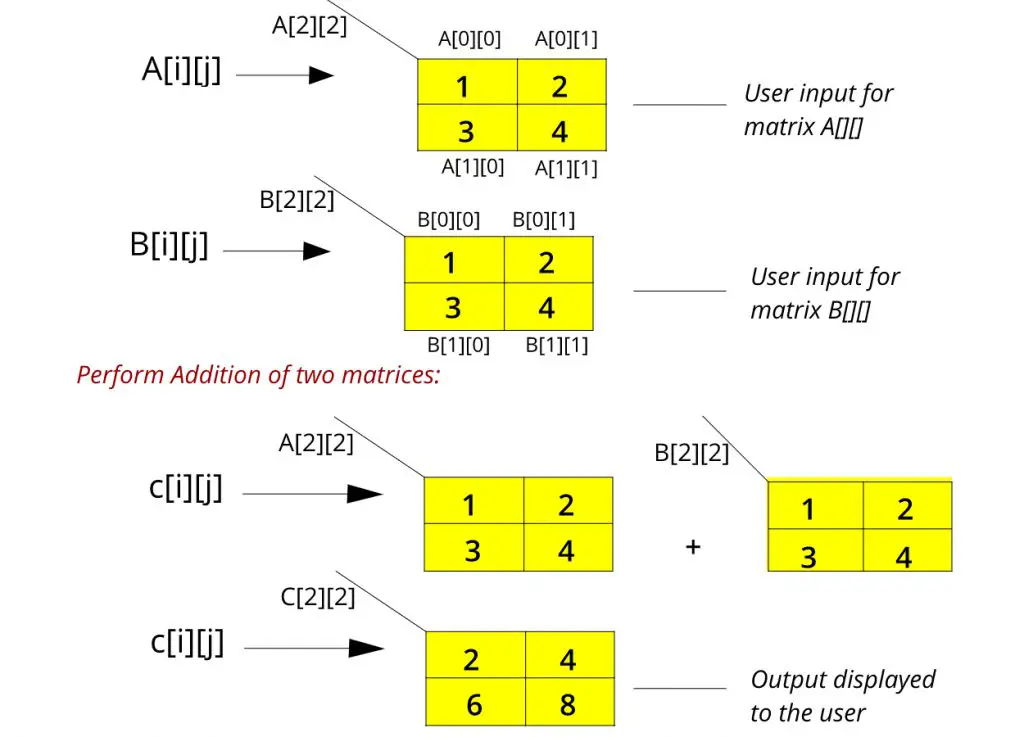
Wrapping up:
Well, I hope it really helped you to know the C++ better.
Attention reader⚠Don’t stop learning now.
Just stay with us to get more such exciting codes to explore more in C++.
View related posts:
- C++ Program to Add Two Matrices (Multi-dimensional Array)
- C++ Program to Add Two Numbers
- C++ Program to Calculate the Simple Interest
- C++ Program to Check Armstrong Number
- C++ Program to Check Palindrome Number
- C++ Program to Check Whether a Character is Vowel or Consonant
- C++ Program to Demonstrate the Arithmetic Operators
- C++ Program to Demonstrate the Assignment Operator
- C++ Program to Display Multi-Dimensional Array
- C++ Program to Display Single Dimensional Array
- C++ Program to Find Factorial of a Number
- C++ Program to Find Out Product of Digits
- C++ Program to Find Out Sum of Digits
- C++ Program to Find Out the Area of Square
- C++ Program to Find the Fibonacci Sequence
- C++ Program to Find the Largest Among Three Numbers
- C++ Program to Reverse a Number
- C++ Program to Swap Two Numbers
- C++ Program to Transpose the Matrix (2-D Array)
Leave a Reply