Like Arithmetic Operator, in this tutorial, we will learn about the Assignment Operators i.e. how to demonstrate the Assignment Operator using C++.
So, hereβs some quick information related to the program which can help you to know better, i.e. What actually it is?
Quick Info:π‘
βͺ Assignment operator is an operator used to assign the value to the variables.
βͺ The basic assignment operator includes:
- += (Combination of β+β and β=β operator)
- -= (Combination of β-β and β=β operator)
- *=(Combination of β*β and β=β operator)
- /= (Combination of β/β and β=β operator)
- %= (Combination of β%β and β=β operator)
βͺ Steps to perform the above task:
- First, input the value of the operands.
- Second, use the different assignment operators to demonstrate it.
- Third, display the result on the output screen.
βͺ It can be done using different ways:
- Using a Simple Method
- Using a Class
- Using a Function
Demonstrate the Assignment Operator Using Simple Method
Hereβs a simple method that demonstrates the Assignment operator.
Example:
#include<iostream.h>
#include<conio.h>
void main()
{
int number;
cout<<"Enter the value of number:";
cin>>number;
//Assigning value by adding 5 to number
number+=5; //number=number+5;
cout<<"Number after += is: "<<number;
//Assigning value by subtracting 5 from number
number-=5; //number=number-5;
cout<<"Number after -= is: "<<number;
//Assigning value by multiplying 5 to number
number*=5; //number=number*5;
cout<<"Number after *= is: "<<number;
//Assigning value by dividing 5 to number
number/=5; //number=number/5;
cout<<"Number after /= is: "<<number;
//Assigning value by dividing 5 to number
number%=5; //number=number%5;
cout<<"Number after %= is: "<<number;
getch();
}
//Output:
Enter the value of number:30
Number after += is: 35
Number after -= is: 30
Number after *= is: 150
Number after /= is: 30
Number after %= is: 0
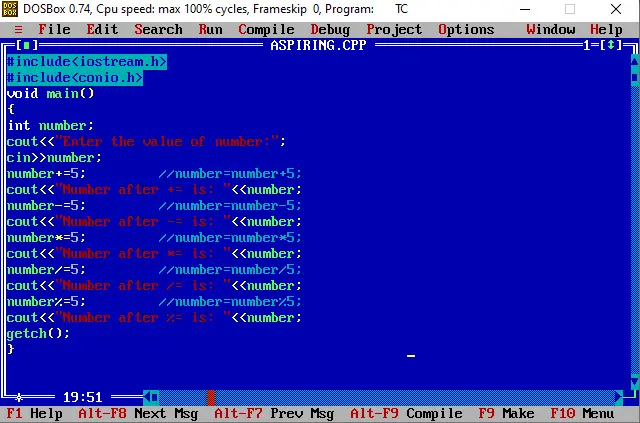
Working:
Flow of a program:
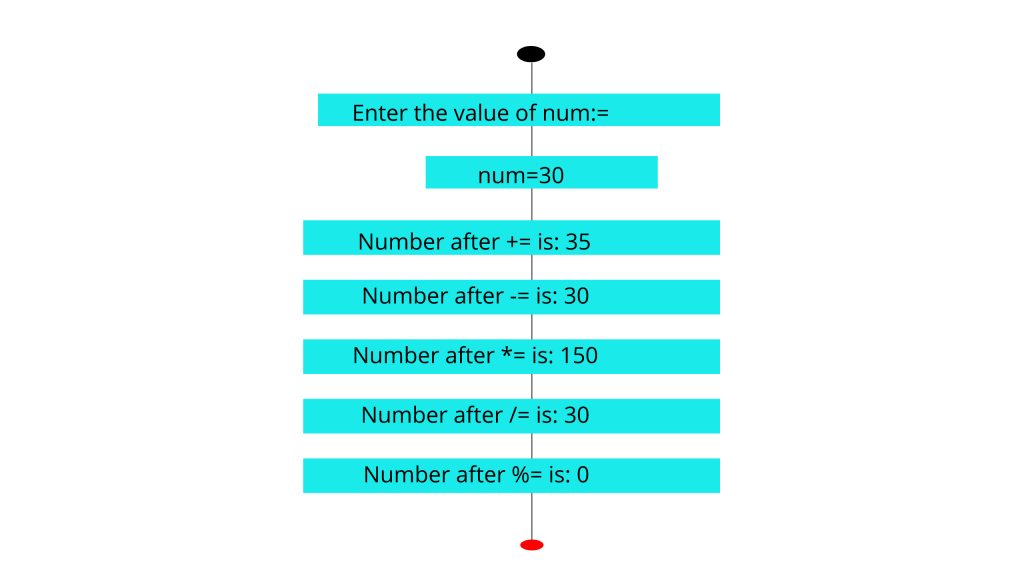
Demonstrate the Assignment Operator Using a Class
A class is a user-defined data type which makes C++ an object-oriented language.
We create a class with two functions getdata and assignment_op. Function getdata is used to get two integers from a user, and function assignment_op operates and displays the result.
So, here I will show you, how to demonstrate the assignment operators using class.
Example:
#include<iostream.h>
#include<conio.h>
class assignment
{
public:
int num;
void getdata()
{
cout<<"Enter the value of num:=";
cin>>num;
}
void assignment_op()
{
cout<<"Number after += is: "<<(num+=5)<<endl;
cout<<"Number after -= is: "<<(num-=5)<<endl;
cout<<"Number after *= is: "<<(num*=5)<<endl;
cout<<"Number after /= is: "<<(num/=5)<<endl;
cout<<"Number after %= is: "<<(num%=5)<<endl;
}
};
void main()
{
assignment obj;
obj.getdata();
obj.assignment_op();
getch();
}
//Output:
Enter the value of number:20
Number after += is: 25
Number after -= is: 20
Number after *= is: 100
Number after /= is: 20
Number after %= is: 0
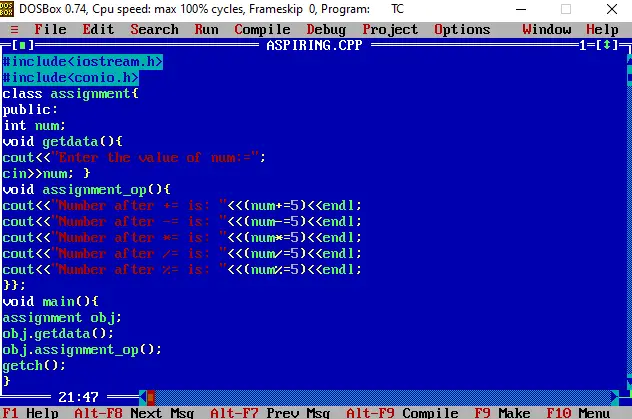
Working:
Flow of a program:
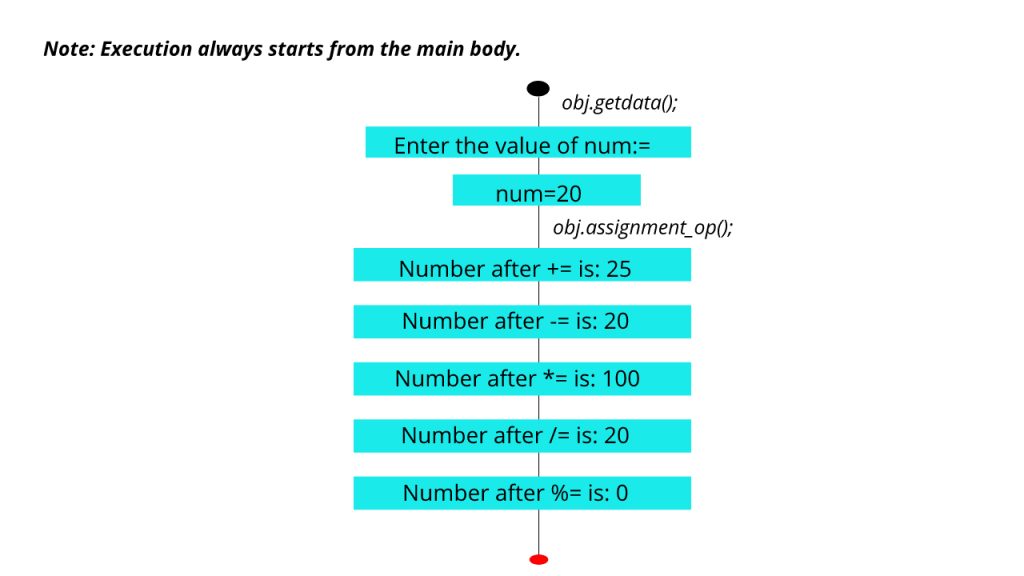
Demonstrate the Assignment Operator Using a Function
A function is a group of statements which perform a specific task.
Actually, it is a self-contained block of statements which performs its task when get executed.
For operations, first, create functions for different assignment operators and pass the arguments for integer.
Example:
#include<iostream.h>
#include<conio.h>
int fun1(int a){
cout<<"Number after += is:=;
return a+=2;
}
int fun2(int a){
cout<<"Number after -= is:=;
return a-=2;
}
int fun3(int a){
cout<<"Number after *= is:=;
return a*=2;
}
int fun4(int a){
cout<<"Number after /= is:=;
return a/=2;
}
int fun5(int a){
cout<<"Number after %= is:=;
return a%=2;
}
void main()
{
int number;
cout<<"Enter the number";
cin>>number;
cout<<fun1(number)<<endl;
cout<<fun2(number)<<endl;
cout<<fun3(number)<<endl;
cout<<fun4(number)<<endl;
cout<<fun5(number)<<endl;
getch();
}
//Output:
Enter the value of number:10
Number after += is: 12
Number after -= is: 8
Number after *= is: 20
Number after /= is: 5
Number after %= is: 0
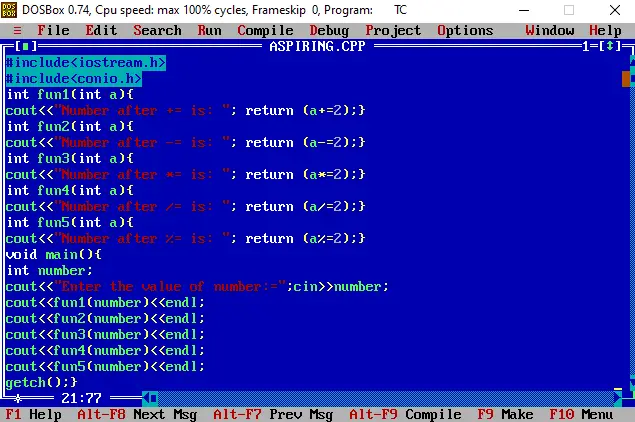
Working:
Flow of a program:
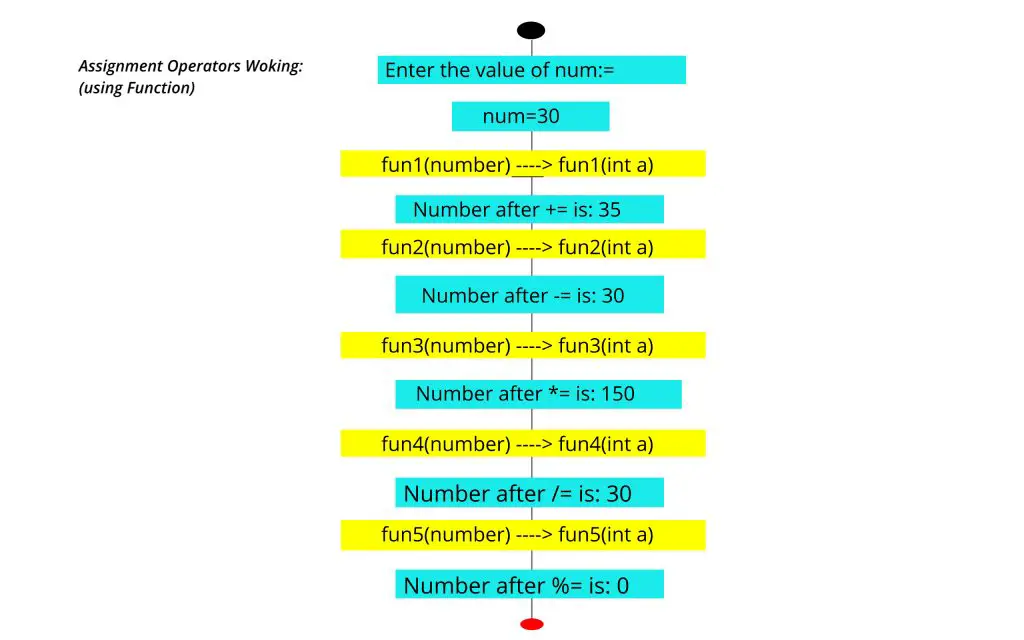
From the above examples, we understood that there are several ways to write a single program using different ways.
Solving a program with different methods is quite interesting. Right?
Well, I hope it really helped you to know C++ better.
And, if you liked this stay with me to get more such exciting codes to explore more in C++.
View related posts:
- C++ Program to Add Two Matrices (Multi-dimensional Array)
- C++ Program to Add Two Numbers
- C++ Program to Calculate the Simple Interest
- C++ Program to Check Armstrong Number
- C++ Program to Check Palindrome Number
- C++ Program to Check Whether a Character is Vowel or Consonant
- C++ Program to Demonstrate the Arithmetic Operators
- C++ Program to Demonstrate the Assignment Operator
- C++ Program to Display Multi-Dimensional Array
- C++ Program to Display Single Dimensional Array
- C++ Program to Find Factorial of a Number
- C++ Program to Find Out Product of Digits
- C++ Program to Find Out Sum of Digits
- C++ Program to Find Out the Area of Square
- C++ Program to Find the Fibonacci Sequence
- C++ Program to Find the Largest Among Three Numbers
- C++ Program to Reverse a Number
- C++ Program to Swap Two Numbers
- C++ Program to Transpose the Matrix (2-D Array)
Leave a Reply