In this tutorial, we will learn about Simple Interest in C++ i.e. how to write a program to calculate the simple interest using C++.
Quick Info:💡
↪ As we know, Simple Interest(S.I) is a quick and easy method of calculating the interest charge on a loan.
↪ For calculating the Simple interest, we require parameters like principal(P), rate(R) and time(T). The formula for calculating Simple Interest is (P*R*T)/100.
↪ Here’s the following steps to calculate the Simple Interest:
- First, initialize the variables- principal, rate, time and SI.
- Second, find the Simple interest using formula.
- Third, print the result of SI on the output console.
Code to calculate simple interest can be performed in two ways:
- By Direct Method
- By Using Function
Program to Calculate Simple Interest Using Direct Method
Here’s a direct method to calculate Simple Interest.
Simply, the user will be asked to enter the values then, the result is computed according to code provided and then displayed to a user.
Example:
#include<iostream.h>
#include<conio.h>
void main()
{
int simple_interest,principal,rate,time;
cout<<"Enter principal";
cin>>principal;
cout<<"Enter rate";
cin>>rate;
cout<<"Enter time";
cin>>time;
simple_interest=(principal*rate*time)/100;
cout<<"Simple Interest is:="<<simple_interest;
getch();
}
//Output:
Enter principal 12000
Enter rate 2
Enter time 2
Simple Interest is:=480
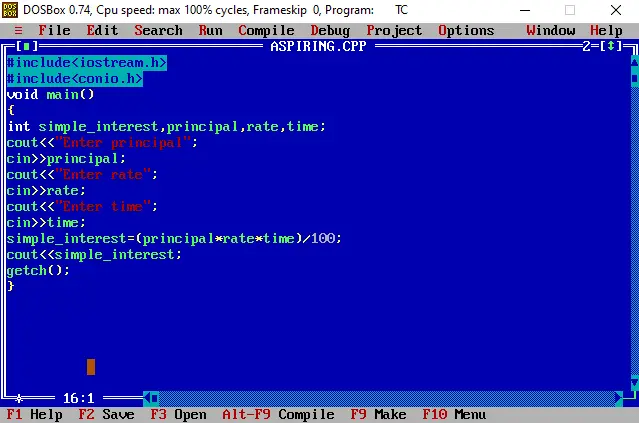
Working:
Flow of the program internally:
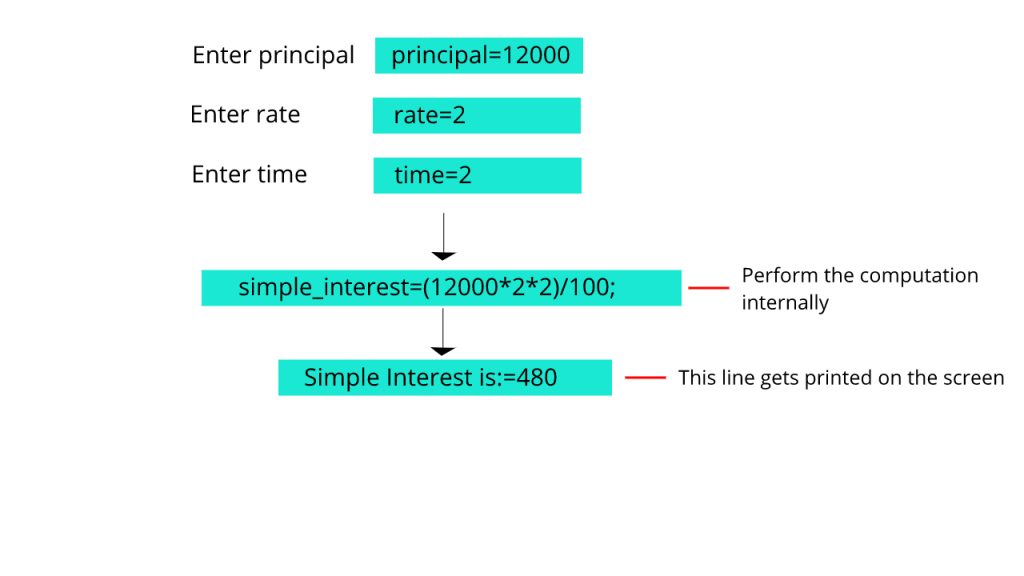
Program to Calculate Simple Interest Using Function
Functions are used to perform certain actions, and they are important for reusing code: Define the code once and use it many times.
And, this is a another method to calculate Simple Interest at large amount i.e. by function.
Note: Always remember that execution starts from the main body only.
Example:
#include<iostream.h>
#include<conio.h>
double SI(double principal, double rate, double time)
{
double simple_interest;
simple_interest=(principal*rate*time)/100;
return simple_interest;
}
double main(){
double SI1=SI(12000,2,2);
double SI2=SI(5500,2,3.5);
cout<<"Simple Interest for SI1 is:="<<SI1<<endl;
cout<<"Simple Interest for SI2 is:="<<SI2<<endl;
getch();
return 0;
}
//Output:
Simple Interest for SI1 is:=480
Simple Interest for SI2 is:=385
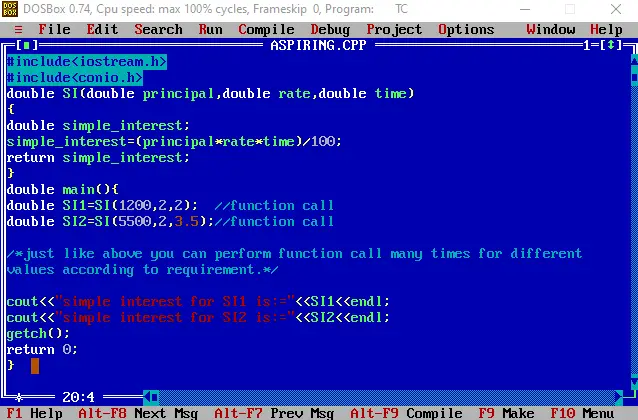
Working:
Flow of a program:
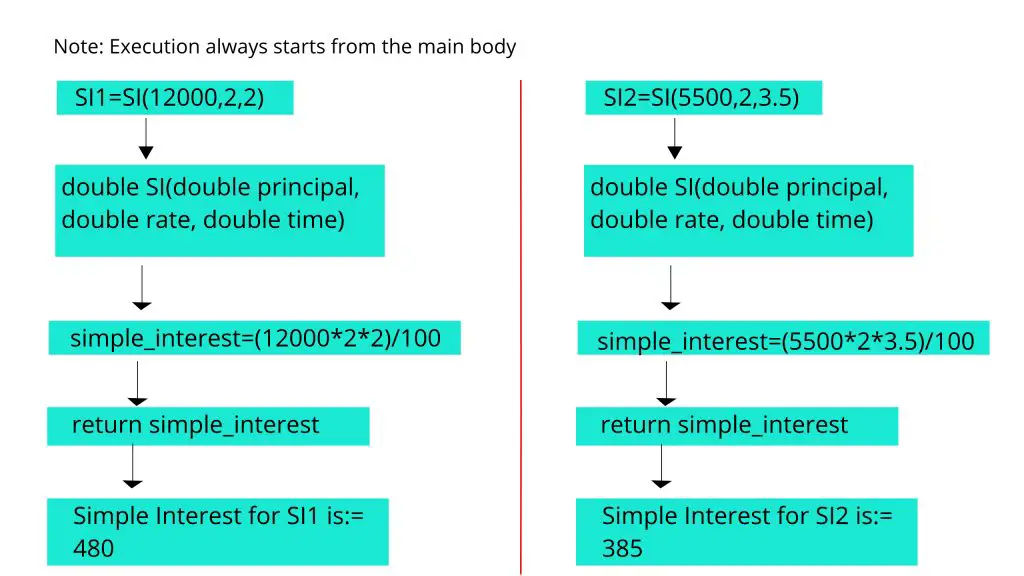
Well, I hope it really helped you to know C++ better.
Attention reader⚠Don’t stop learning now.
Just stay with us to get more such exciting codes to explore more in C++.
View related posts:
- C++ Program to Add Two Matrices (Multi-dimensional Array)
- C++ Program to Add Two Numbers
- C++ Program to Calculate the Simple Interest
- C++ Program to Check Armstrong Number
- C++ Program to Check Palindrome Number
- C++ Program to Check Whether a Character is Vowel or Consonant
- C++ Program to Demonstrate the Arithmetic Operators
- C++ Program to Demonstrate the Assignment Operator
- C++ Program to Display Multi-Dimensional Array
- C++ Program to Display Single Dimensional Array
- C++ Program to Find Factorial of a Number
- C++ Program to Find Out Product of Digits
- C++ Program to Find Out Sum of Digits
- C++ Program to Find Out the Area of Square
- C++ Program to Find the Fibonacci Sequence
- C++ Program to Find the Largest Among Three Numbers
- C++ Program to Reverse a Number
- C++ Program to Swap Two Numbers
- C++ Program to Transpose the Matrix (2-D Array)
Leave a Reply