In this tutorial, we will learn about factorial in C++ i.e. how to write a program to find factorial of a number using C++.
Quick Info:💡
↪ Factorial of a number is the product of all the integers from 1 to that number.
↪ For example, the factorial of 4 (denoted by 4!) is 1*2*3*4 i.e. 24. Factorial of a negative number is not defined and the factorial of 0! is 1.
↪ Here, we will find the factorial of a number to display its output on the output console.
Factorial of a number can be performed in two ways:
- Without Recursion
- With Recursion
Factorial of a Number Without Recursion
Factorial of a number without recursion is a simple program to find it.
Simply, the user will be asked to enter a positive integer then, the factorial of a number is computed and displayed to a user.
Example:
#include<iostream.h>
#include<conio.h>
void main(){
int num;
long fact=1;
cout<<"Enter a number:=";
cin>>num;
if(num<0)
cout<<"Factorial of a negative number is not defined";
if(n==0)
cout<<"Factorial of zero is 1";
else
{
while(num>0)
{
fact=fact*num;
num--;
}
cout<<"Factorial:="<<fact;
}
getch();
}
//Output:
Enter a number:=5
Factorial:=120
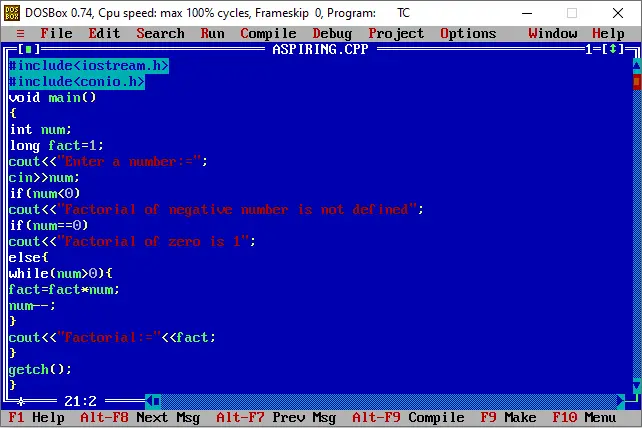
Working: (Factorial without recursion)
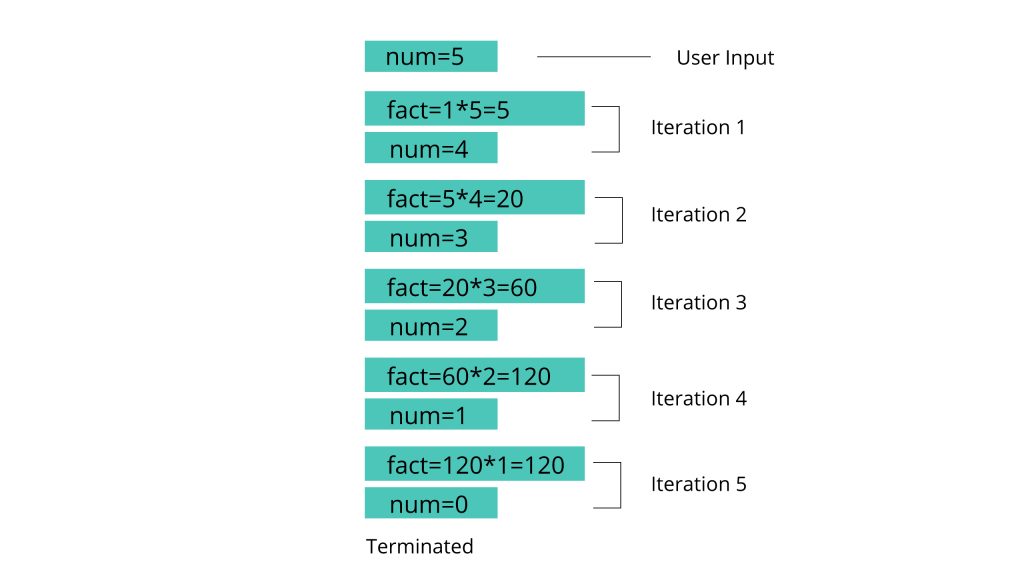
Factorial of a Number With Recursion
Recursion (Recursive function) is defined as – the function calls itself repeatedly unless the code included in the body gets terminated.
Note: Always remember that execution starts from the main body only.
Example:
#include<iostream.h>
#include<conio.h>
int fact(int n)
{
if (n==0)||(n==1)
return 1;
else
return fact(n-1)*n;
}
void main(){
int n;
cout<<"enter a positive integer:=";
cin>>n;
cout<<"Factorial is:="<<fact(n);
getch();
}
//Output:
enter a positive integer:=4
Factorial is:=24
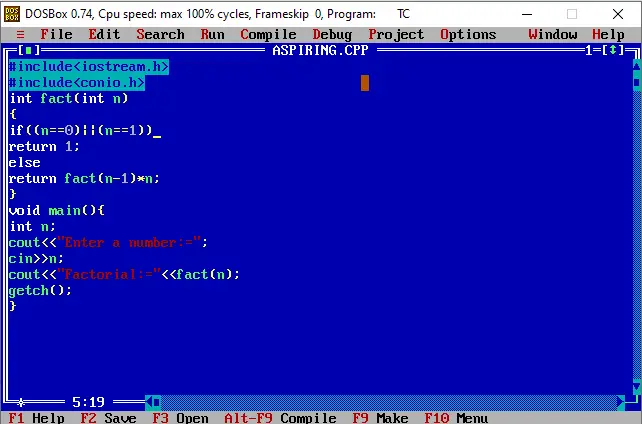
Working: (Factorial with Recursion)
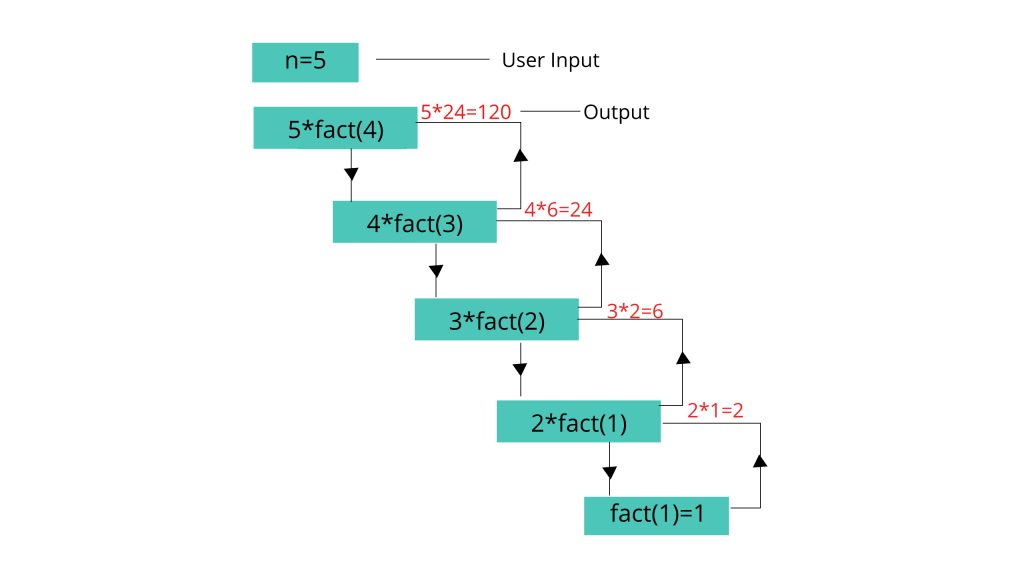
Note: Due to recursion, for each function call, an entry is first created in the stack frame and are then executed in a LIFO manner(i.e. Last In First Out).
Want more programs on recursion?
Just Visit: Fibonacci,
Well, I hope it really helped you to know C++ better.
Attention reader⚠Don’t stop learning now.
Just stay with us to get more such exciting codes to explore more in C++.
View related posts:
- C++ Program to Add Two Matrices (Multi-dimensional Array)
- C++ Program to Add Two Numbers
- C++ Program to Calculate the Simple Interest
- C++ Program to Check Armstrong Number
- C++ Program to Check Palindrome Number
- C++ Program to Check Whether a Character is Vowel or Consonant
- C++ Program to Demonstrate the Arithmetic Operators
- C++ Program to Demonstrate the Assignment Operator
- C++ Program to Display Multi-Dimensional Array
- C++ Program to Display Single Dimensional Array
- C++ Program to Find Factorial of a Number
- C++ Program to Find Out Product of Digits
- C++ Program to Find Out Sum of Digits
- C++ Program to Find Out the Area of Square
- C++ Program to Find the Fibonacci Sequence
- C++ Program to Find the Largest Among Three Numbers
- C++ Program to Reverse a Number
- C++ Program to Swap Two Numbers
- C++ Program to Transpose the Matrix (2-D Array)
Leave a Reply