In this tutorial, we will learn about the product of digits in C++ i.e. how to write a program to find out the product of digits of any number using C++.
So, here’s some quick information related to the program which can help you to know the topic. i.e, What actually it says?
Quick Info:💡
↪ A program to compute the product of digits in an integer.
↪ For example, 345=3*4*5=60
↪ Steps to find out the product of digits:
- Get any number by the user
- Get the remainder of the number
- Find the product of a remainder
- Divide the number by 10
- Repeat above steps till number becomes less than 0
Find Out Product of Digits Using While loop
For finding the product of digits, we will try to execute the above steps with the help of C++ programming language.
Here’s a direct and simple method to find out product of digits.
Simply, the user will be asked to enter an integer then, the number is computed according to code provided and then the result is displayed to a user.
Example:
#include<iostream.h>
#include<conio.h>
void main()
{
int num, prod=1, rem;
cout<<"Enter any number:=";
cin>>num;
while(num>0)
{
rem=num%10;
prod=prod*rem;
num=num/10;
}
cout<<"Product of digits:="<<product;
getch();
}
//Output:
Enter any number:=1213
Product of digits:=6
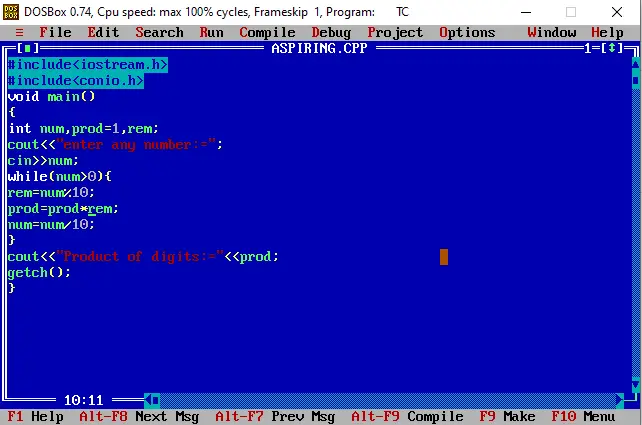
Working:
Flow of a Program:
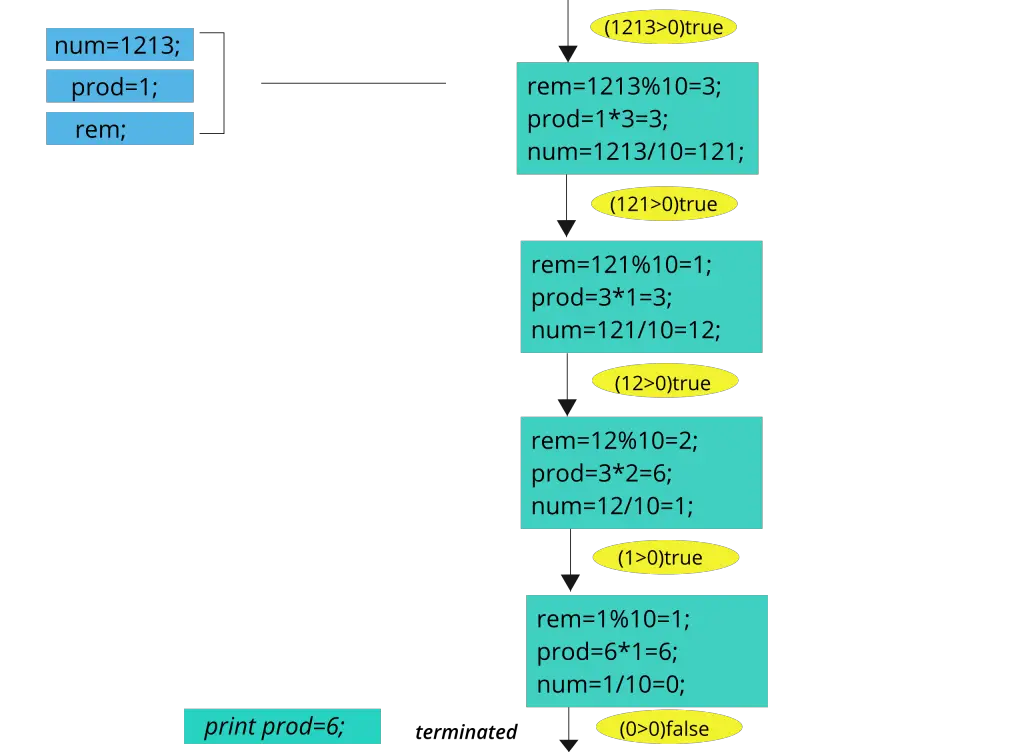
Above figure is the working explanation of the program.
And, you can also try this program(i.e. the product of digits) using the function as discussed below.
Find Out Product of Digits Using Function
Functions are used to perform certain actions, and they are important for reusing code: Define the code once and use it many times.
And, this is a another method to find out the product of digits in an integer i.e. by function.
Note: Always remember that execution starts from the main body only.
Example:
#include<iostream.h>
#include<conio.h>
int prod(int num)
{
int prod=1,rem;
while(num>0)
{
rem=num%10;
prod=prod*rem;
num=num/10;
}
cout<<"Product of digits:="<<prod;
return 0;}
int main(){
int n;
cout<<"Enter any number:=";
cin>>n;
prod(n);
getch();
return 0;
}
//Output:
Enter any number:=1223
Product of digits:=12
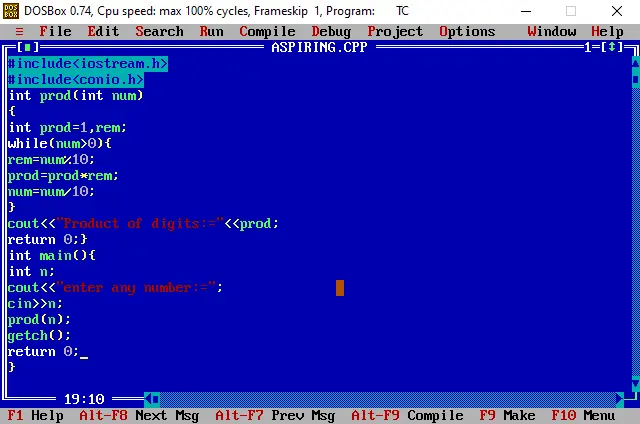
Working:
Flow of a program:
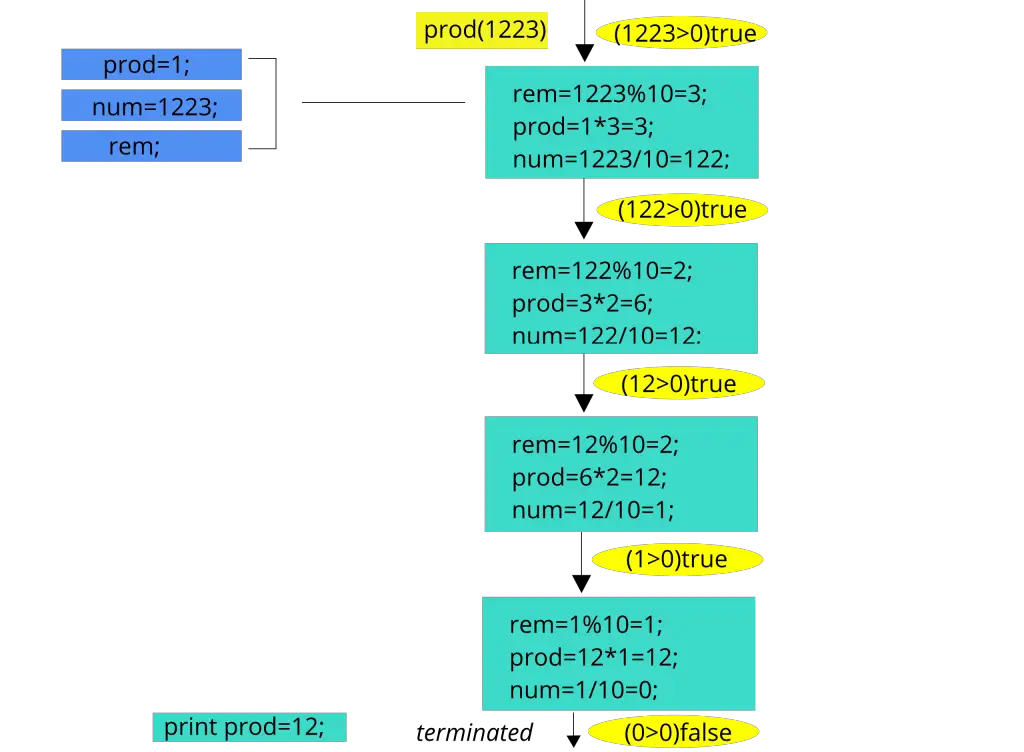
Well, I hope it really helped you to know the C++ better.
Attention reader⚠ Don’t stop learning now.
Let’s try more programs similar to above codes like sum of digits etc.
Just stay with us to get more such exciting codes to explore more in C++.
View related posts:
- C++ Program to Add Two Matrices (Multi-dimensional Array)
- C++ Program to Add Two Numbers
- C++ Program to Calculate the Simple Interest
- C++ Program to Check Armstrong Number
- C++ Program to Check Palindrome Number
- C++ Program to Check Whether a Character is Vowel or Consonant
- C++ Program to Demonstrate the Arithmetic Operators
- C++ Program to Demonstrate the Assignment Operator
- C++ Program to Display Multi-Dimensional Array
- C++ Program to Display Single Dimensional Array
- C++ Program to Find Factorial of a Number
- C++ Program to Find Out Product of Digits
- C++ Program to Find Out Sum of Digits
- C++ Program to Find Out the Area of Square
- C++ Program to Find the Fibonacci Sequence
- C++ Program to Find the Largest Among Three Numbers
- C++ Program to Reverse a Number
- C++ Program to Swap Two Numbers
- C++ Program to Transpose the Matrix (2-D Array)
Leave a Reply