In this tutorial, we will learn about the Arithmetic Operator i.e. how to demonstrate the Arithmetic Operators using C++
So, here’s some quick information related to the program which can help you to know better, i.e. What actually it says?
Quick Info:💡
↪ Arithmetic Operator is a binary operator used to perform tasks on the operands.
↪The basic arithmetic operators include :
- Addition (+)
- Subtraction (-)
- Multiplication (*)
- Division (/)
- Modulus (%)
↪ Steps to perform the above task:
- First, Input the value of the operands.
- Second, Use the different arithmetic operators to demonstrate it.
- Third, Display the result on the output screen.
It can be done using different ways:
- Using a Simple Method
- Using a Class
- Using a Function
Program to Demonstrate the Arithmetic Operators Using Simple Method
Here’s a simple method that demonstrates the Arithmetic operations.
Example:
#include<iostream.h>
#include<conio.h>
void main()
{
int a,b;
cout<<"Enter the value of a:";
cin>>a;
cout<<"Enter the value of b:";
cin>>b;
int sum=a+b;
cout<<"Addition is:="<<sum<<endl;
int sub=a-b;
cout<<"Subtraction is:="<<sub<<endl;
int mul=a*b;
cout<<"Multiplication is:="<<mul<<endl;
int div=a/b;
cout<<"Division is:="<<div<<endl;
int mod=a%b;
cout<<"Modulus is:="<<mod<<endl;
getch();
}
//Output:
Enter the value of a:10
Enter the value of b:5
Addition is:=15
Subtraction is:=5
Multiplication is:=50
Division is:=2
Modulus is:=0
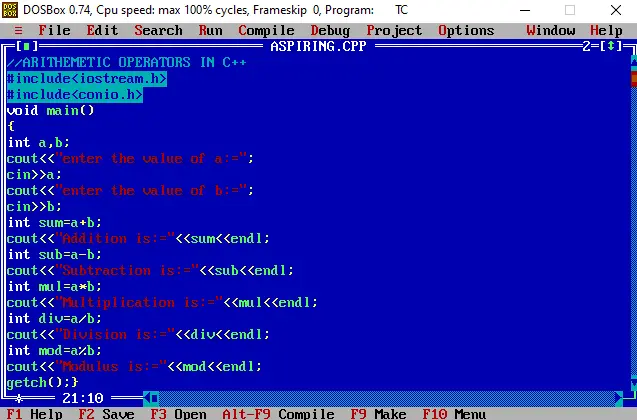
Working:
Flow of a program:
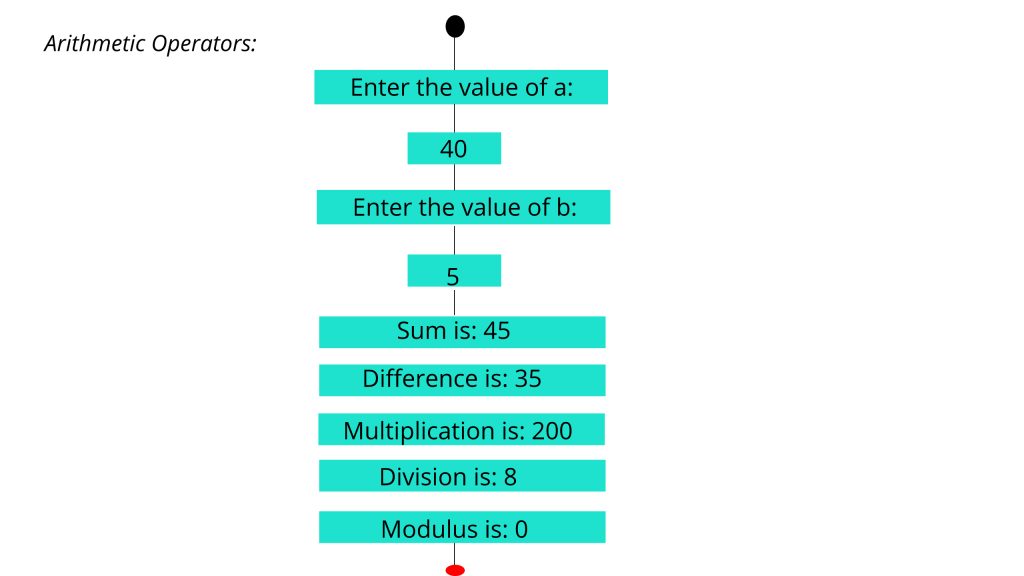
Program to Demonstrate the Arithmetic Operators Using Class
A class is a user-defined data type which makes C++ an object-oriented language.
We create a class with two functions getdata and arithmetic_op. Function getdata is used to get two integers from a user, and function arithmetic_op operates and displays the result.
So, here I will show you, how to demonstrate the arithmetic operators using class.
Example:
#include<iostream.h>
#include<conio.h>
class arithmetic
{
public:
int a, b;
void getdata()
{
cout<<"Enter the value of a:=";
cin>>a;
cout<<"Enter the value of b:=";
cin>>b;
}
void arithmetic_op()
{
cout<<"Sum is:="<<(a+b)<<endl;
cout<<"Difference is:="<<(a-b)<<endl;
cout<<"Multiplication is:="<<(a*b)<<endl;
cout<<"Division is:="<<(a/b)<<endl;
cout<<"Modulus is:="<<(a%b)<<endl;
}
};
void main()
{
arithmetic obj;
obj.getdata();
obj.arithmetic_op();
getch();
}
//Output:
Enter the value of a:20
Enter the value of b:5
Sum is:=25
Subtraction is:=15
Multiplication is:=100
Division is:=4
Modulus is:=0
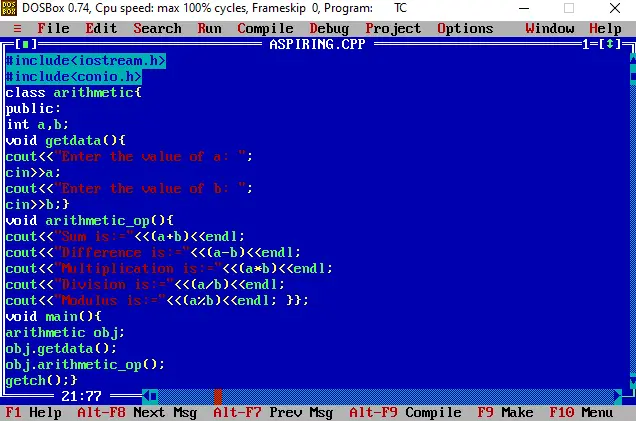
Working:
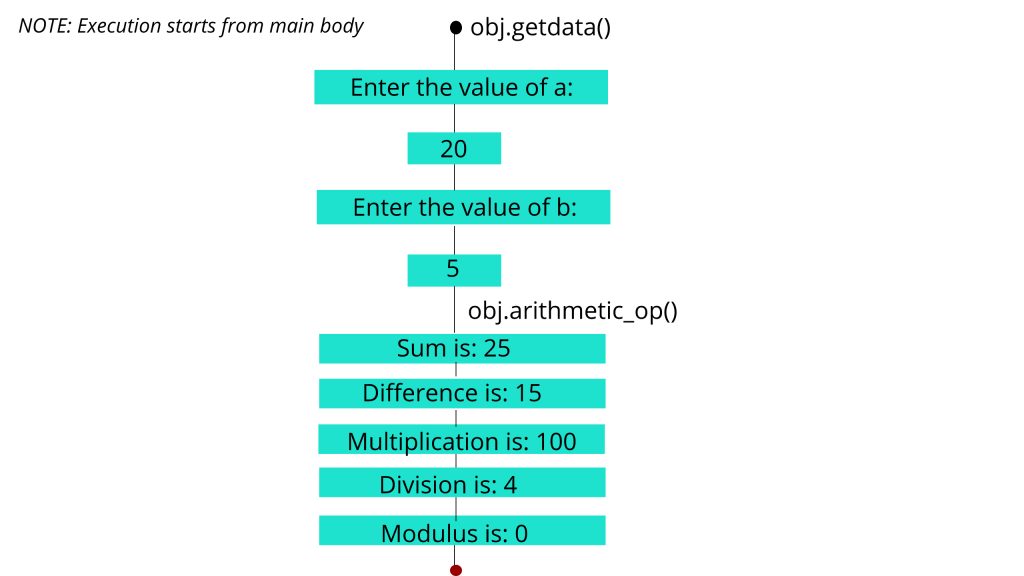
Program to Demonstrate the Arithmetic Operators Using Function
A function is a group of statements which perform a specific task.
Actually, it is a self-contained block of statements which performs its task when get executed.
For operations, first create functions for different arithmetic operations and pass the arguments for two integers.
Example:
#include<iostream.h>
#include<conio.h>
int add(int a, int b){
cout<<"Addition is: ";
return (a+b);
}
int sub(int a, int b){
cout<<"Subtraction is: ";
return (a-b);
}
int mul(int a, int b){
cout<<"Multiplication is: ";
return (a*b);
}
int div(int a, int b){
cout<<"Division is: ";
return (a/b);
}
int mod(int a, int b){
cout<<"Modulus is: ";
return (a%b);
}
void main()
{
int first_no,second_no;
cout<<"Enter the first number:";
cin>>first_no;
cout<<"Enter the second number:";
cin>>second_no;
cout<<add(first_no, second_no)<<endl;
cout<<sub(first_no, second_no)<<endl;
cout<<mul(first_no, second_no)<<endl;
cout<<div(first_no, second_no)<<endl;
cout<<mod(first_no, second_no)<<endl;
getch();
}
//Output:
Enter the first number:30
Enter the second number:5
Addition is:=35
Subtraction is:=25
Multiplication is:=150
Division is:=6
Modulus is:=0
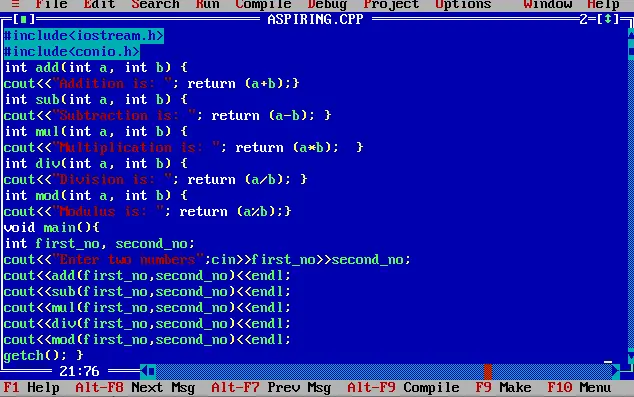
Working:
Flow of a program:
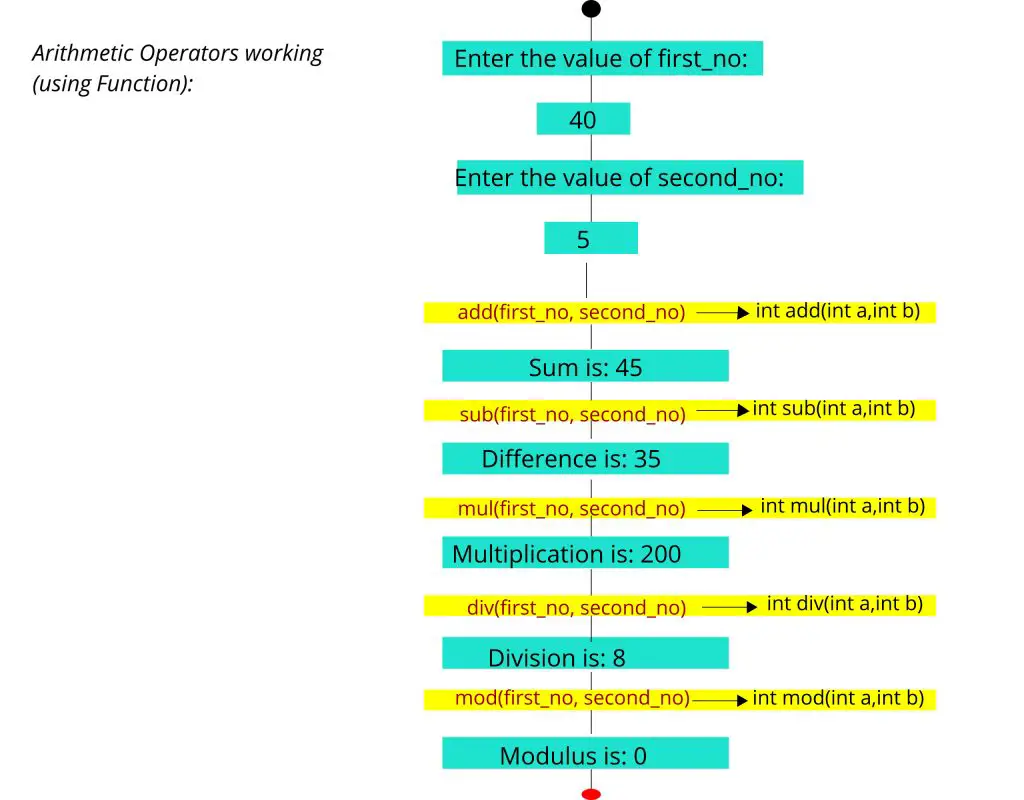
Wrapping Up:
From the above examples, we understood that there are several ways to write a single program using different ways.
Sounds Interesting! Right?
Well, I hope it really helped you to know C++ better.
And, if you liked this stay with us to get more such exciting codes to explore more in C++.
View related posts:
- C++ Program to Add Two Matrices (Multi-dimensional Array)
- C++ Program to Add Two Numbers
- C++ Program to Calculate the Simple Interest
- C++ Program to Check Armstrong Number
- C++ Program to Check Palindrome Number
- C++ Program to Check Whether a Character is Vowel or Consonant
- C++ Program to Demonstrate the Arithmetic Operators
- C++ Program to Demonstrate the Assignment Operator
- C++ Program to Display Multi-Dimensional Array
- C++ Program to Display Single Dimensional Array
- C++ Program to Find Factorial of a Number
- C++ Program to Find Out Product of Digits
- C++ Program to Find Out Sum of Digits
- C++ Program to Find Out the Area of Square
- C++ Program to Find the Fibonacci Sequence
- C++ Program to Find the Largest Among Three Numbers
- C++ Program to Reverse a Number
- C++ Program to Swap Two Numbers
- C++ Program to Transpose the Matrix (2-D Array)
Leave a Reply