In this tutorial, we will learn about the swapping of numbers in C++ i.e. how to write a program to swap two numbers using C++.
Quick Info:💡
↪ Swapping of two numbers means exchange the values of two variables with each other.
↪ For example, suppose you have a two numbers var1=45 and var2=50 then, after swapping numbers will become var1=50 and var2=45.
↪ Here’s the following steps to swap the number:
- First, consider any two digits number.
- Second, assign the first value to the second variable.
- Third, assign the second value to the first variable.
↪ Here’s the two methods to perform the swapping of two numbers:
- Without using third variable
- With using third variable
Program to Swap Two Numbers Without Using Third Variable
Swapping of two numbers without using a third variable(i.e. temporary variable) is the simplest way to swap the numbers.
Steps to perform above task:
- Initially, take two variables (a and b).
- Second, add two variables and store it in the first variable(i.e. a).
- Third, subtract two variables and store it in a second variable(i.e. b).
- Again, subtract two variables and store it in the first variable(i.e. a)
Example:
#include<iostream.h>
#include<conio.h>
void main()
{
int first_number, second_number;
cout<<"Enter first number";
cin>>first_number;
cout<<"Enter second number";
cin>>second_number;
first_number=first_number+second_number;
second_number=first_number-second_number;
first_number=first_number-second_number;
cout<<"Numbers after swapping:="<<endl;
cout<<"First number after swapping: "<<first_number<<endl;
cout<<"Second number after swapping: "<<second_number<<endl;
getch();
}
//Output:
Enter first number 34
Enter second number 67
Numbers after swapping:=
First number after swapping: 67
Second number after swapping: 34
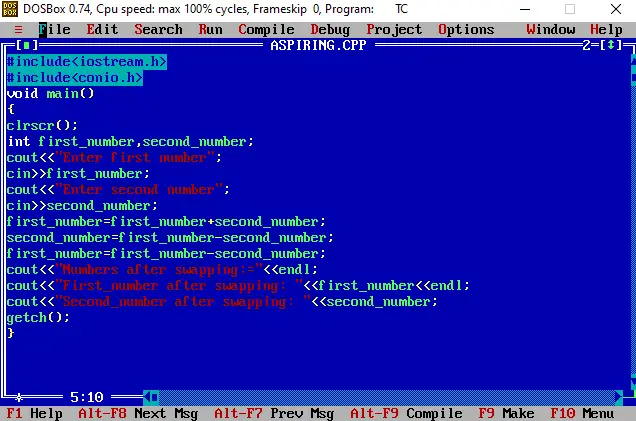
Working:
Flow of the program:
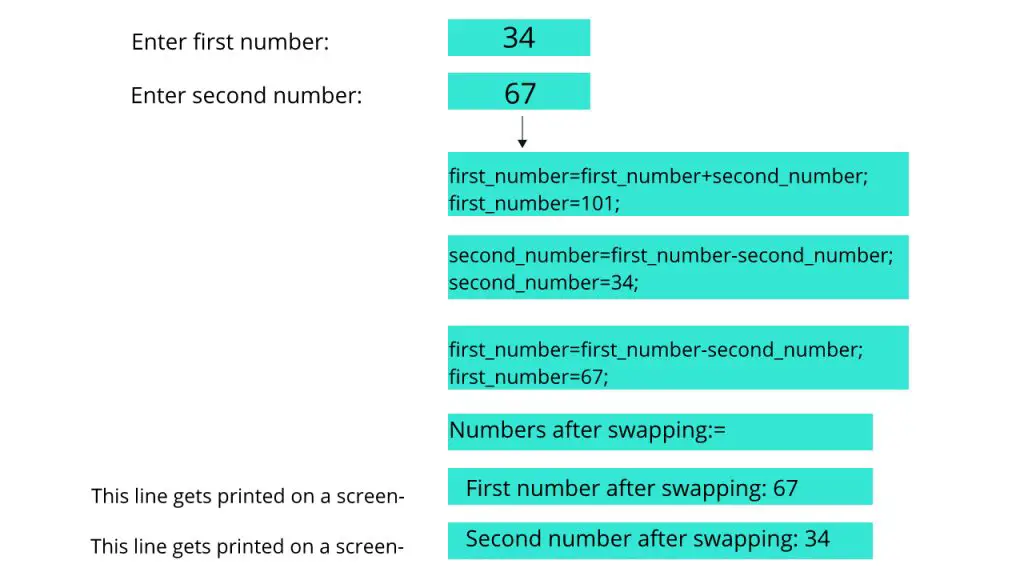
Program to Swap Two Numbers Using Third Variable
Another way to swap two numbers is using a temporary variable as a third variable.
Steps to perform above tasks:
- User is asked to enter the values (a and b).
- Assign the value of the first variable to the temporary variable.
- Assign the value of the second variable to the first variable.
- Assign the value of the third variable to the second variable.
Example:
#include<iostream.h>
#include<conio.h>
void main()
{
int first_number, second_number, temp;
cout<<"Enter first number";
cin>>first_number;
cout<<"Enter second number";
cin>>second_number;
temp=first_number;
first_number=second_number;
second_number=temp;
cout<<"Numbers after swapping are:="<<endl;
cout<<"First number after swapping: "<<first_number<<endl;
cout<<"Second number after swapping: "<<second_number;
getch();
}
//Output:
Enter first number 34
Enter second number 67
Numbers after swapping are:=
First number after swapping: 67
Second number after swapping: 34
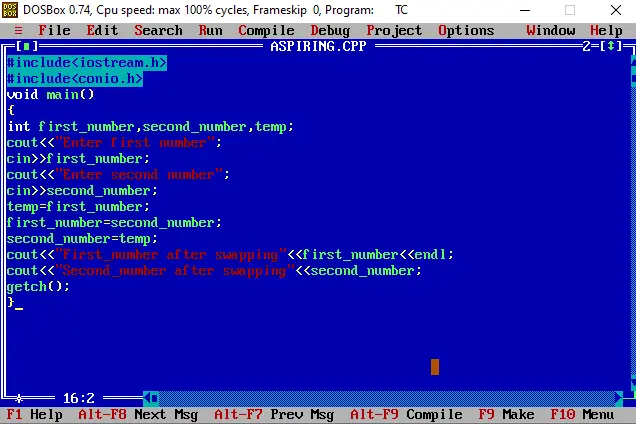
Working:
Flow of the program:
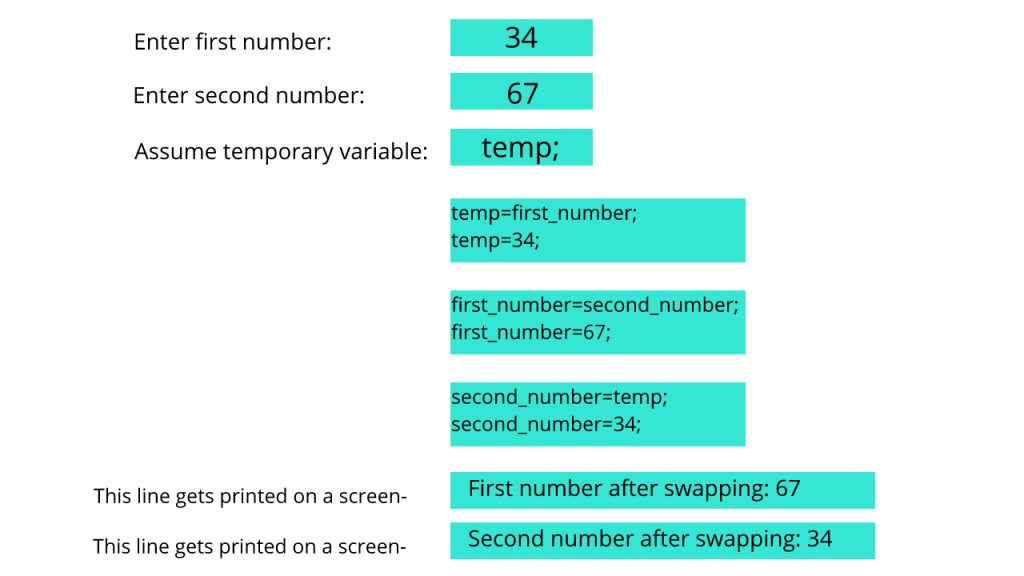
Wrapping Up:
From the above examples, we understood that there are several ways to write a single program using different ways.
Sounds Interesting! Right?
Well, I hope it really helped you to know C++ better.
And, if you liked this stay with us to get more such exciting codes to explore more in C++.
View related posts:
- C++ Program to Add Two Matrices (Multi-dimensional Array)
- C++ Program to Add Two Numbers
- C++ Program to Calculate the Simple Interest
- C++ Program to Check Armstrong Number
- C++ Program to Check Palindrome Number
- C++ Program to Check Whether a Character is Vowel or Consonant
- C++ Program to Demonstrate the Arithmetic Operators
- C++ Program to Demonstrate the Assignment Operator
- C++ Program to Display Multi-Dimensional Array
- C++ Program to Display Single Dimensional Array
- C++ Program to Find Factorial of a Number
- C++ Program to Find Out Product of Digits
- C++ Program to Find Out Sum of Digits
- C++ Program to Find Out the Area of Square
- C++ Program to Find the Fibonacci Sequence
- C++ Program to Find the Largest Among Three Numbers
- C++ Program to Reverse a Number
- C++ Program to Swap Two Numbers
- C++ Program to Transpose the Matrix (2-D Array)
Leave a Reply