In this tutorial, we will learn about Armstrong numbers in C++ i.e. how to write a program to check whether a number is Armstrong or not using C++.
Quick Info:💡
↪ An Armstrong number of a three-digit number is the sum of cubes of its digit is equal to the number itself.
For Example, 371=> 33+73+13=27+343+1=371
                       153=> 13+53+33 =1+125+27=153 …etc
↪ Here, we will write a code to check whether a number is armstrong or not and display its output on the output console.
Code to check armstrong number can be performed in two ways:
- Simple Method
- By Function
Check Armstrong Number Using Simple Method
Here’s a direct method to check an armstrong number.
Simply, the user will be asked to enter a three-digit positive integer then, the number is computed and displayed to a user.
Example:
#include<iostream.h>
#include<conio.h>
void main()
{
int num,sum=0,rem,temp;
cout<<"enter any three digits number:=";
cin>>num;
temp=num;
while(temp>0)
{
rem=temp%10;
temp=temp/10;
sum=sum+rem*rem*rem;
}
if(sum==num)
cout<<"Number is Armstrong";
else
cout<<"Number is not Armstrong";
getch();
}
//Output:
enter any three digits number:=371
Number is Armstrong
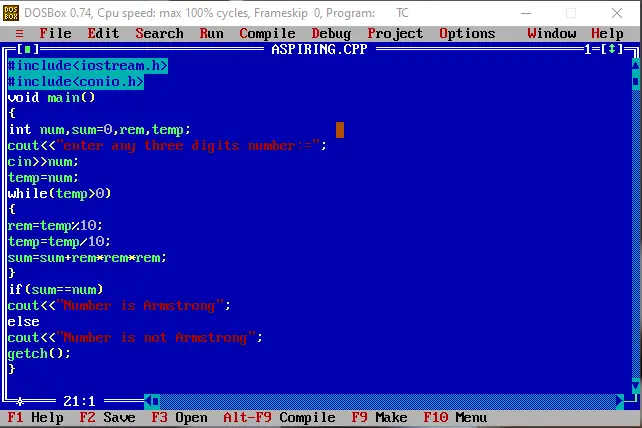
Working:
Flow of a program:
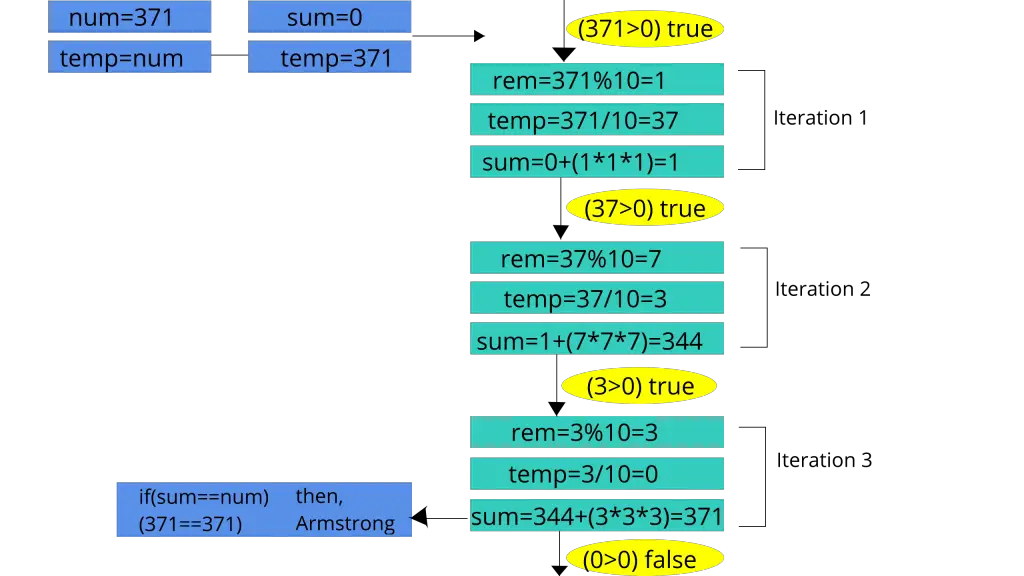
Check Armstrong Number Using Function
Functions are used to perform certain actions, and they are important for reusing code: Define the code once and use it many times.
And, this is a another method to check whether it is armstrong or not i.e. by function
Note: Always remember that execution starts from the main body only.
Example:
#include<iostream.h>
#include<conio.h>
int armstrong(int num)
{
int sum=0,rem,temp;
temp=num;
while(temp>0)
{
rem=temp%10;
temp=temp/10;
sum=sum+rem*rem*rem;
}
if(sum==num)
cout<<"Number is Armstrong";
else
cout<<"Number is not Armstrong";
return 0;
int main(){
int n;
cout<<"enter any three digits number:=";
cin>>n;
armstrong(n);
getch();
return 0;
}
//Output:
enter any three digits number:=153
Number is Armstrong
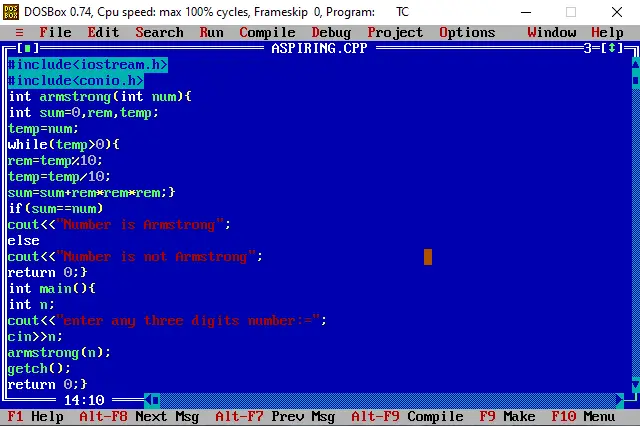
Working:
Flow of a program:
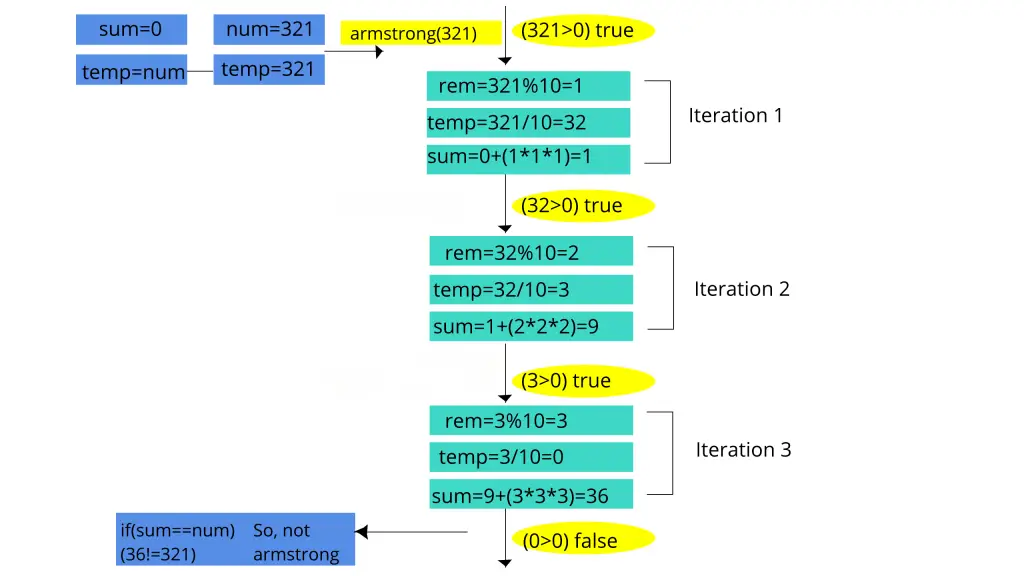
Well, I hope it really helped you to know C++ better.
Attention reader⚠Don’t stop learning now.
Like Armstrong Number, check whether a number is a palindrome or not is also an important program in C++.
Just stay with us to get more such exciting codes to explore more in C++.
View related posts:
- C++ Program to Add Two Matrices (Multi-dimensional Array)
- C++ Program to Add Two Numbers
- C++ Program to Calculate the Simple Interest
- C++ Program to Check Armstrong Number
- C++ Program to Check Palindrome Number
- C++ Program to Check Whether a Character is Vowel or Consonant
- C++ Program to Demonstrate the Arithmetic Operators
- C++ Program to Demonstrate the Assignment Operator
- C++ Program to Display Multi-Dimensional Array
- C++ Program to Display Single Dimensional Array
- C++ Program to Find Factorial of a Number
- C++ Program to Find Out Product of Digits
- C++ Program to Find Out Sum of Digits
- C++ Program to Find Out the Area of Square
- C++ Program to Find the Fibonacci Sequence
- C++ Program to Find the Largest Among Three Numbers
- C++ Program to Reverse a Number
- C++ Program to Swap Two Numbers
- C++ Program to Transpose the Matrix (2-D Array)
Leave a Reply