In this tutorial, we will learn about Palindrome numbers in C++ i.e. how to write a program to check whether a number is a Palindrome or not using C++.
Quick Info:💡
↪ An integer is considered as Palindrome if the reverse of the number is equal to its original number.
↪ For example, 121, 343, 1221, etc are the palindrome numbers.
↪ Here’s the following steps to check palindrome:
- First, consider any number.
- Second, find the reverse of the number
- Third, check whether the reverse is equal to the number or not.
Code to check palindrome number can be performed in two ways:
- Direct method
- By Function
Palindrome Number in C++
Here’s a direct method to check a palindrome number.
Simply, the user will be asked to enter an integer then, the number is computed according to code provided and then displayed to a user.
Example:
#include<iostream.h>
#include<conio.h>
void main()
{
int num,rev=0,rem,temp;
cout<<"enter a number:=";
cin>>num;
temp=num;
while(temp>0)
{
rem=temp%10;
rev=rev*10+rem;
temp/=10;
}
cout<<"Number was:"<<num<<endl;
cout<<"Reverse is:"<<rev<<endl;
if(num==rev)
cout<<"So, number is Palindrome";
else
cout<<"So, number is not Palindrome";
getch();
}
//Output:
Enter a number 343
Number was: 343
Reverse is: 343
So, number is Palindrome
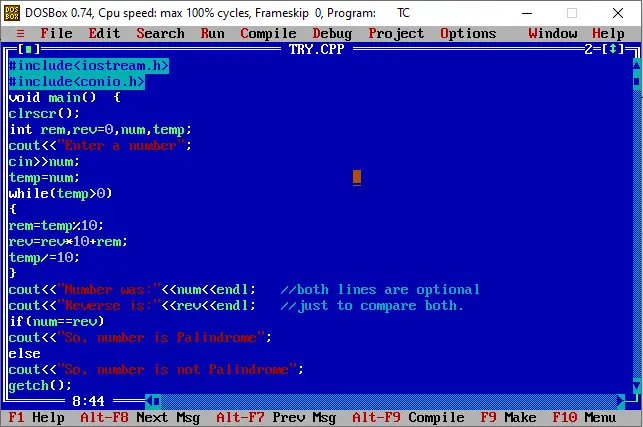
Working:
Flow of a program:
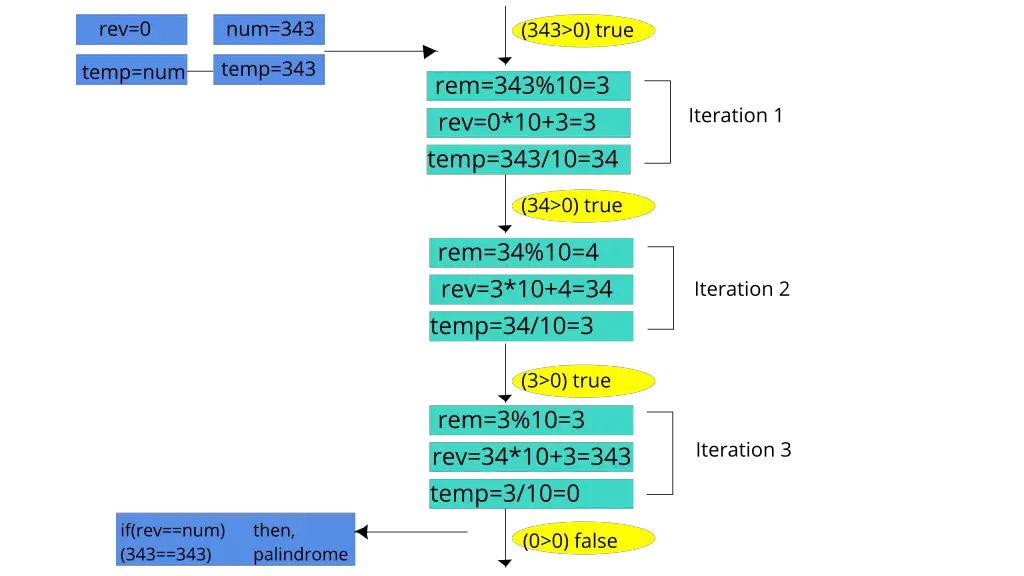
Palindrome Number Using Function
Functions are used to perform certain actions, and they are important for reusing code: Define the code once and use it many times.
And, this is a another method to check whether it is palindrome or not i.e. by function
Note: Always remember that execution starts from the main body only.
Example:
#include<iostream.h>
#include<conio.h>
int palindrome(int num)
{
int rev=0,rem,temp;
temp=num;
while(temp>0)
{
rem=temp%10;
rev=rev*10+rem;
temp=temp/10;
}
if(rev==num)
cout<<"Number is Palindrome";
else
cout<<"Number is not Palindrome";
return 0;}
int main(){
int n;
cout<<"enter any number:=";
cin>>n;
palindrome(n);
getch();
return 0;
}
//Output:
enter any number:=345
Number is not Palindrome
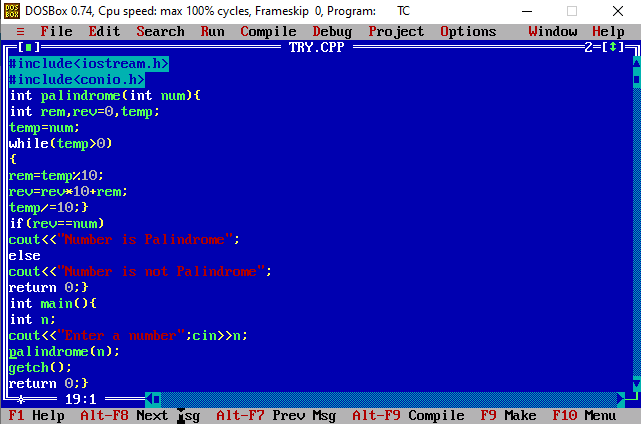
Working:
Flow of a program:
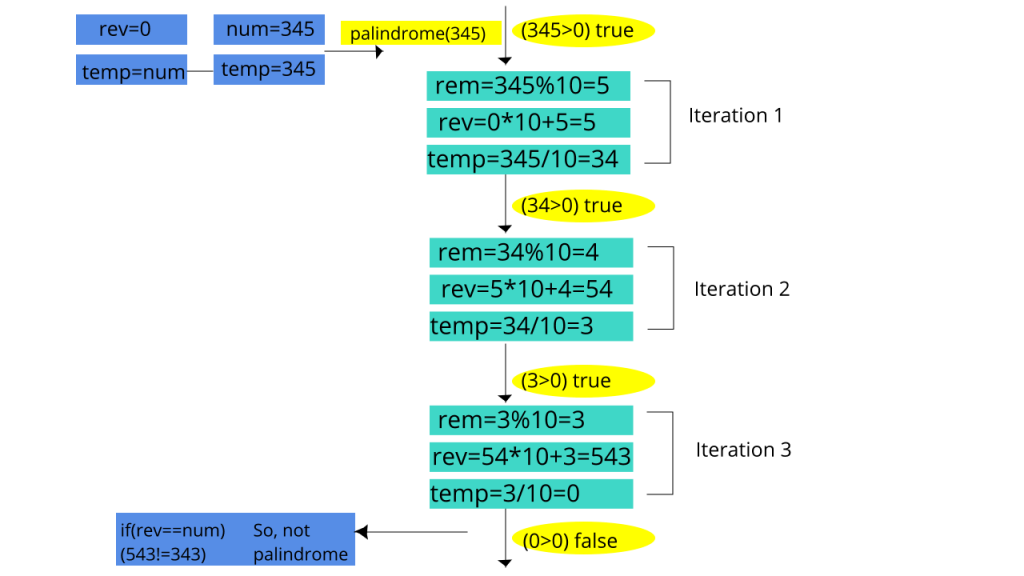
Well, I hope it really helped you to know C++ better.
Attention reader⚠Don’t stop learning now.
Just stay with us to get more such exciting codes to explore more in C++.
View related posts:
- C++ Program to Add Two Matrices (Multi-dimensional Array)
- C++ Program to Add Two Numbers
- C++ Program to Calculate the Simple Interest
- C++ Program to Check Armstrong Number
- C++ Program to Check Palindrome Number
- C++ Program to Check Whether a Character is Vowel or Consonant
- C++ Program to Demonstrate the Arithmetic Operators
- C++ Program to Demonstrate the Assignment Operator
- C++ Program to Display Multi-Dimensional Array
- C++ Program to Display Single Dimensional Array
- C++ Program to Find Factorial of a Number
- C++ Program to Find Out Product of Digits
- C++ Program to Find Out Sum of Digits
- C++ Program to Find Out the Area of Square
- C++ Program to Find the Fibonacci Sequence
- C++ Program to Find the Largest Among Three Numbers
- C++ Program to Reverse a Number
- C++ Program to Swap Two Numbers
- C++ Program to Transpose the Matrix (2-D Array)
Leave a Reply