In this tutorial, we will learn to check whether a character is vowel or consonant using C++.
So, here’s some quick information related to the program which can help you to know better. i.e, What actually says?
Quick Info:💡
↪ It’s a program to check whether an alphabet is vowel or consonant.
↪ Five alphabets a, e, i, o and u are known as vowels. All other alphabets except these 5 letters are known as consonants.
↪ If the alphabet is a vowel, it will print “Vowel” as the output on the output console but, if it is a consonant, it will print “Consonant” as the output on the output console.
↪ It can be done using different ways:
- By using an if-else.
- By using an if-else ladder
- By using a switch case
↪ Steps to check whether an alphabet is vowel or consonant:
- First, Get any character by the user.
- Apply the conditions according to the syntax of if-else/switch case/if-else ladder as your choice.
- Print the result on the output console.
Check Whether a Character is Vowel or Consonant Using If-Else
If-else is a conditional statement that executes the code depending on the true or false.
In the If-else statement, a block of code is executed if the condition is evaluated true and another block of code is executed if the condition is false.
Syntax:
if(conditional expression)
<statement to be executed if condition is true>
else
<statement to be executed if condition is false>
Example:
#include<iostream.h>
#include<conio.h>
void main()
{
char alpha;
cout<<"Enter an alphabet:=";
cin>>alpha;
if(alpha=='a'||alpha=='e'||alpha=='i'||alpha=='o'||alpha=='u')
cout<<"It is vowel";
else
cout<<"It is consonant";
getch();
}
//Output:
Enter an alphabet:=r
It is consonant
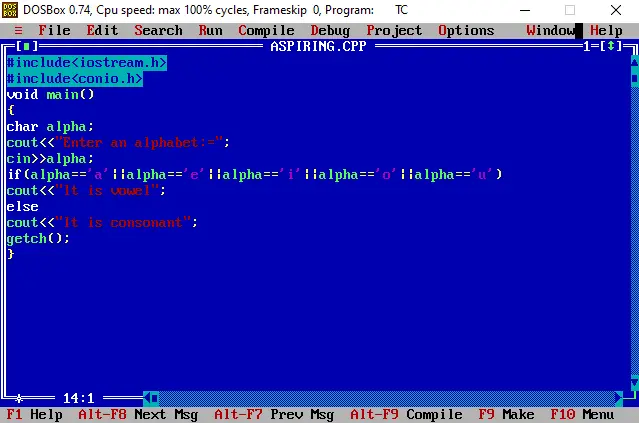
Working:
Flow of a program:
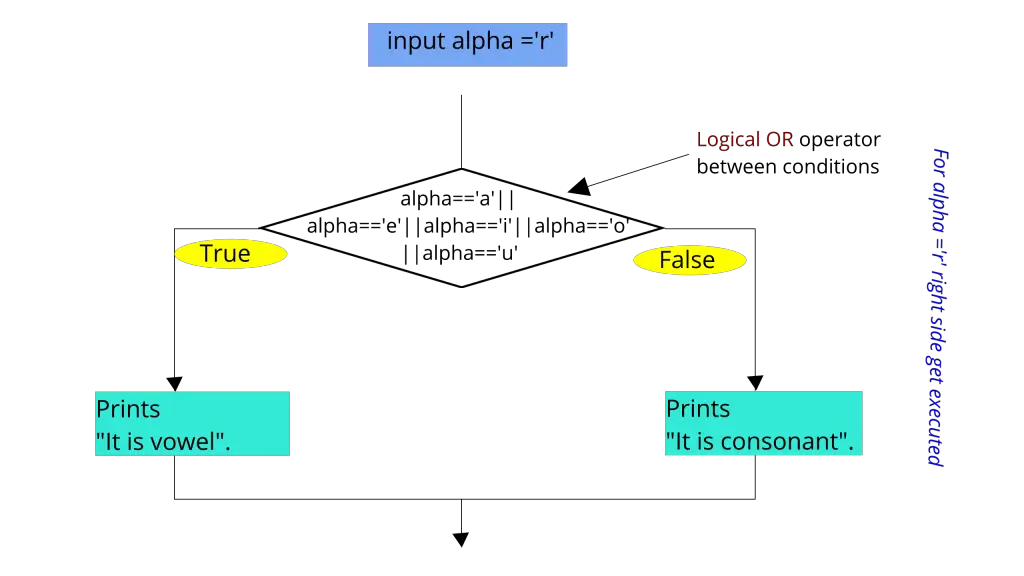
Here, according to user input alpha = ‘r’ so, the condition becomes ‘false’ and right side get executed.
Check Whether a Character is Vowel or Consonant Using If-Else Ladder
An entire sequence of the if-else statements occur one after another is called as an if-else ladder.
Syntax:
if(conditional expression)
<statement>
else if(conditional expression)
<statement>
else if(conditional expression)
<statement>
....
Example:
#include<iostream.h>
#include<conio.h>
void main()
{
char alpha;
cout<<"Enter an alphabet:=";
cin>>alpha;
if(alpha=='a')
cout<<"It is vowel";
else if(alpha=='e')
cout<<"It is vowel";
else if(alpha=='i')
cout<<"It is vowel";
else if(alpha=='o')
cout<<"It is vowel";
else if(alpha=='u')
cout<<"It is vowel";
else
cout<<"It is consonant";
getch();
}
//Output:
Enter an alphabet:=o
It is vowel
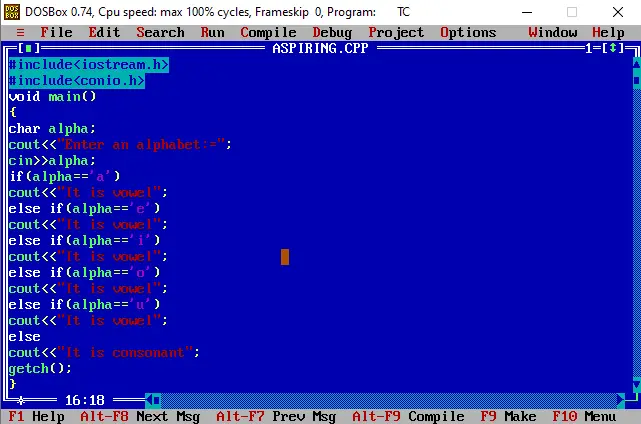
Woking:
Flow of a program:
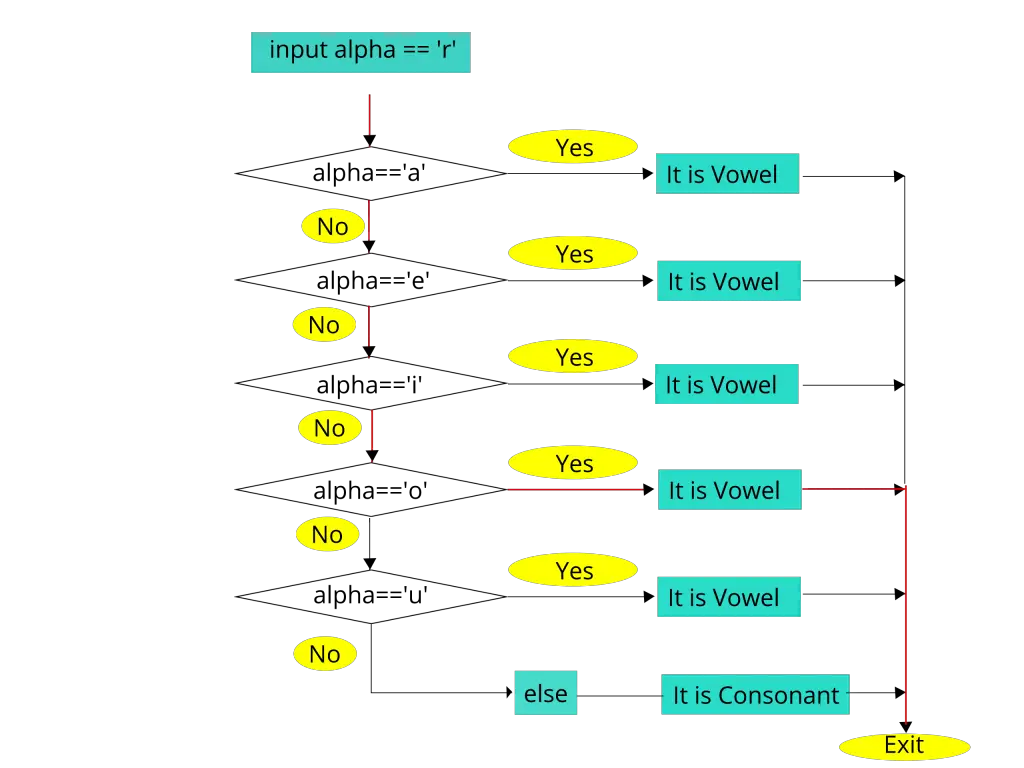
Check Whether a Character is Vowel or Consonant Using Switch-Case
A switch statement is a conditional statement that tests a value against different values.
If the value is matched, the corresponding group of statement is executed. If not then default statement is executed.
A switch statement begins with the ‘switch’ keyword followed by a value expression in the () parenthesis. Each case is separated by the break statement.
Syntax:
switch(expression)
{
case value1: statement;
break;
case value2: statement;
break;
.......
default: default statement;
}
Example:
#include<iostream.h>
#include<conio.h>
void main()
{
char alpha;
cout<<"Enter an alphabet:=";
cin>>alpha;
switch(alpha)
{
case 'a':
cout<<"It is vowel";
break;
case 'e':
cout<<"It is vowel";
break;
case 'i':
cout<<"It is vowel";
break;
case 'o':
cout<<"It is vowel";
break;
case 'u':
cout<<"It is vowel";
break;
default:
cout<<"It is consonant";
}
getch();
}
//Output:
Enter an alphabet:=i
It is vowel
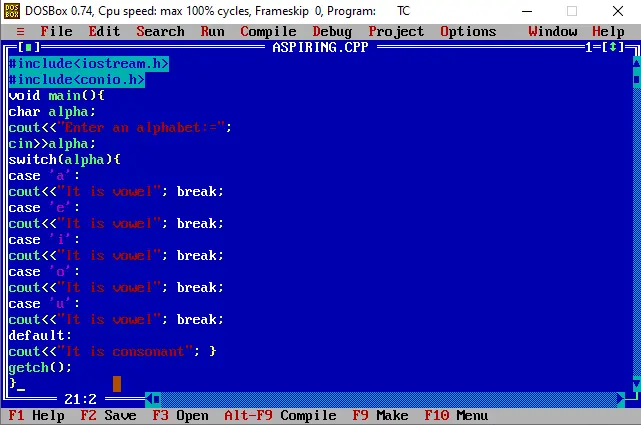
Working:
Flow of a program:
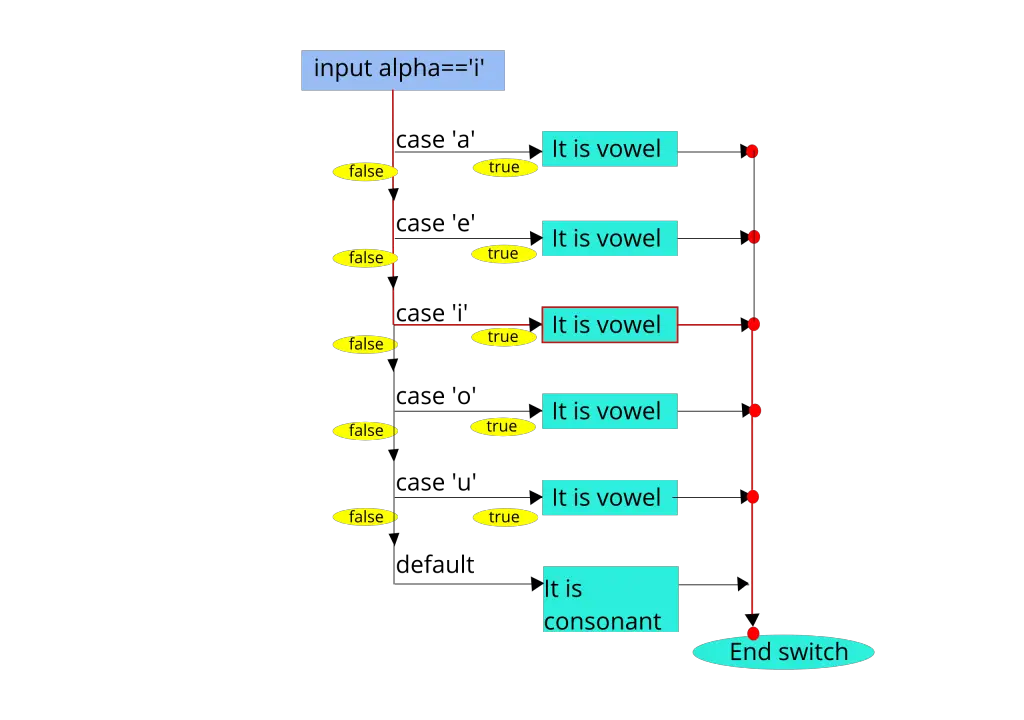
Well, I hope it really helped you to know C++ better.
Attention reader⚠ Don’t stop learning now.
You must try this program using class in free time, one of the class examples is addition using class.
Just stay with us to get more such exciting codes to explore more in C++.
View related posts:
- C++ Program to Add Two Matrices (Multi-dimensional Array)
- C++ Program to Add Two Numbers
- C++ Program to Calculate the Simple Interest
- C++ Program to Check Armstrong Number
- C++ Program to Check Palindrome Number
- C++ Program to Check Whether a Character is Vowel or Consonant
- C++ Program to Demonstrate the Arithmetic Operators
- C++ Program to Demonstrate the Assignment Operator
- C++ Program to Display Multi-Dimensional Array
- C++ Program to Display Single Dimensional Array
- C++ Program to Find Factorial of a Number
- C++ Program to Find Out Product of Digits
- C++ Program to Find Out Sum of Digits
- C++ Program to Find Out the Area of Square
- C++ Program to Find the Fibonacci Sequence
- C++ Program to Find the Largest Among Three Numbers
- C++ Program to Reverse a Number
- C++ Program to Swap Two Numbers
- C++ Program to Transpose the Matrix (2-D Array)
Leave a Reply