In this tutorial, we’ll learn about the transpose of matrices (2-D Array) i.e. a program to transpose the matrix using the C++ programming language.
Quick Info:💡
↪ Transpose of matrix means exchanging the rows into column and column into rows.
↪ For example, suppose you have a matrix of [3][3] {(1 2 3),(4 5 6),(7 8 9)} then, the result after transpose of the matrix will be [3][3] {(1 4 7),(2 5 8),(3 6 9)}.
↪ Steps to perform above task:
- First, input the square matrix.
- Second, perform the transpose of matrix i.e. transpose[i][j]==matrix[j][i].
- Third, display the result on the output screen.
Program to Transpose the Matrix (2-D Array)
Example:
#include<iostream.h>
#include<conio.h>
void main()
{
int matrix[20][20],row,col,i,j,transpose[20][20];
cout<<"Enter number of rows of matrix:=";
cin>>row;
cout<<"Enter number of column of matrix:=";
cin>>col;
if(row==col){
cout<<"Enter the elements of array:"<<endl;
for(i=0;i<row;i++){
for(j=0;j<col;j++){
cout<<"Enter the elements of matrix["<<i<<"]["<<j<<"]:";
cin>>matrix[i][j];
}
}
cout<<"Matrix before transposition:";
for(i=0;i<row;i++){
for(j=0;j<col;j++){
cout<<matrix[i][j]<<"\t";
}
cout<<"\n";
}
cout<<"Matrix after transposition:";
for(i=0;i<row;i++){
for(j=0;j<col;j++){
transpose[i][j]=matrix[j][i];
cout<<transpose[i][j]<<"\t";
}
cout<<"\n";
}}
else
cout<<"transpose not possible";
getch();
}
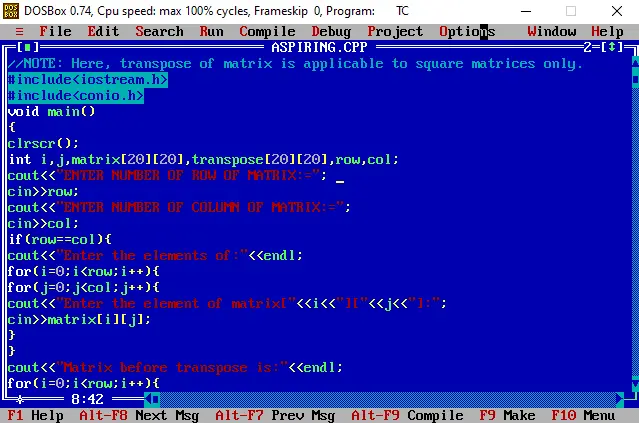
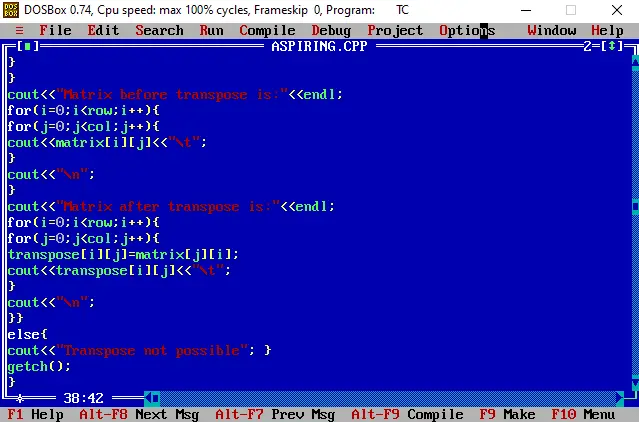
Output:
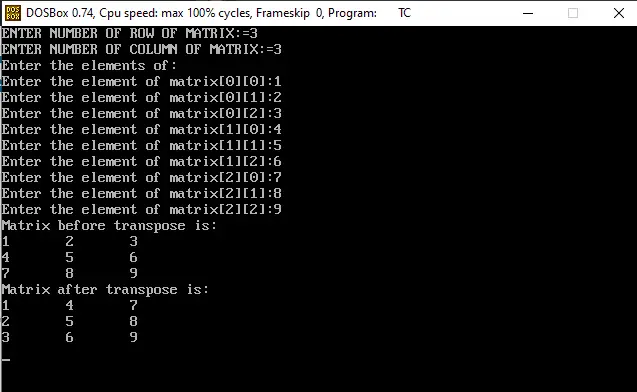
Working:
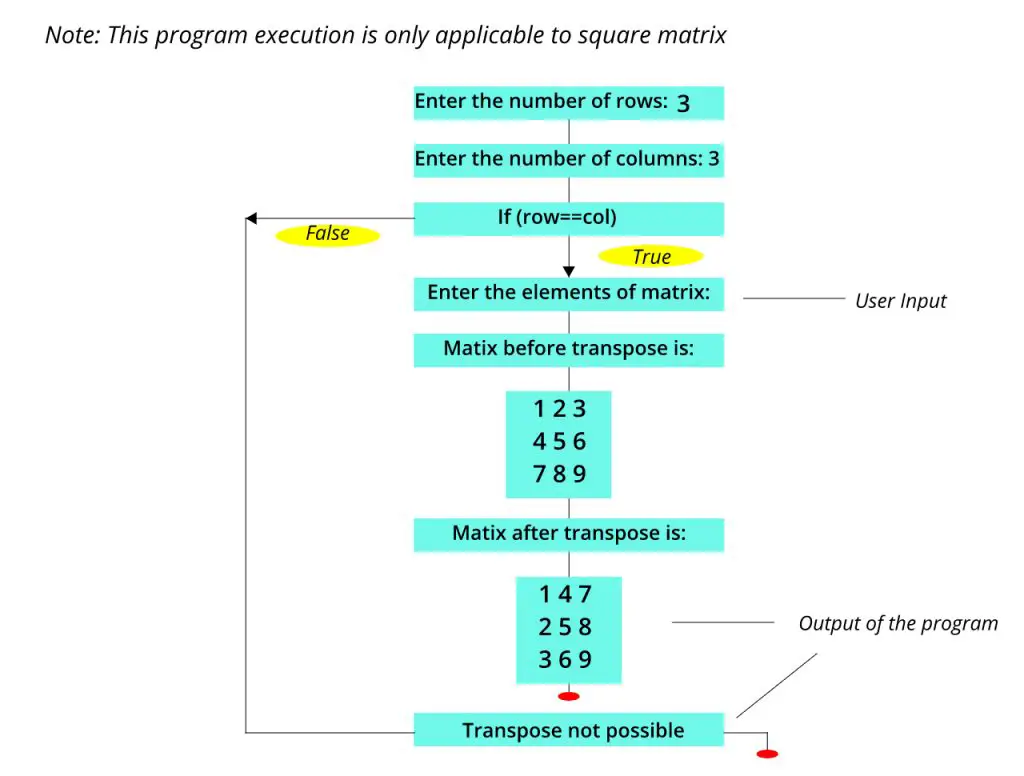
Wrapping up:
Well, I hope it really helped you to know C++ better.
Attention reader⚠ Don’t stop learning now.
Just stay with us to get more such exciting codes to explore more in C++.
View related posts:
- C++ Program to Add Two Matrices (Multi-dimensional Array)
- C++ Program to Add Two Numbers
- C++ Program to Calculate the Simple Interest
- C++ Program to Check Armstrong Number
- C++ Program to Check Palindrome Number
- C++ Program to Check Whether a Character is Vowel or Consonant
- C++ Program to Demonstrate the Arithmetic Operators
- C++ Program to Demonstrate the Assignment Operator
- C++ Program to Display Multi-Dimensional Array
- C++ Program to Display Single Dimensional Array
- C++ Program to Find Factorial of a Number
- C++ Program to Find Out Product of Digits
- C++ Program to Find Out Sum of Digits
- C++ Program to Find Out the Area of Square
- C++ Program to Find the Fibonacci Sequence
- C++ Program to Find the Largest Among Three Numbers
- C++ Program to Reverse a Number
- C++ Program to Swap Two Numbers
- C++ Program to Transpose the Matrix (2-D Array)
Leave a Reply