In this tutorial, we will learn about the area of circle i.e. how to find out the area of circle using C++
So, here’s some quick information related to the program which can help you to know better, i.e. What actually it says?
Quick Info:💡
↪ Circle is a round-shaped figure with no edges or corners i.e. circle contains parameters such as radius, and diameter.
↪ We provide you with the radius of the circle and your task is to display the result as the area of the square on the output console.
↪ Steps to perform the above task:
- First, Input the radius of a circle.
- Second, Use the formula to find out the area i.e. Area = 3.14*radius*radius
- Third, Display the result on the output screen.
↪ It can be done using different ways:
- Using a direct method
- Using function
Find Out Area of Circle Using Direct Method
Here’s a direct method to print the output on the output screen.
Simply, the user will be asked to enter the input and the area of a circle is computed and displayed to the user.
Example:
#include<iostream.h>
#include<conio.h>
void main()
{
double radius, area;
cout<<"Enter the radius of circle: ";
cin>>radius;
area=3.14*radius*radius;
cout<<"Area of circle is: ";
cout<<area;
getch();
}
//Output:
Enter the radius of circle: 5
Area of circle is: 78.5
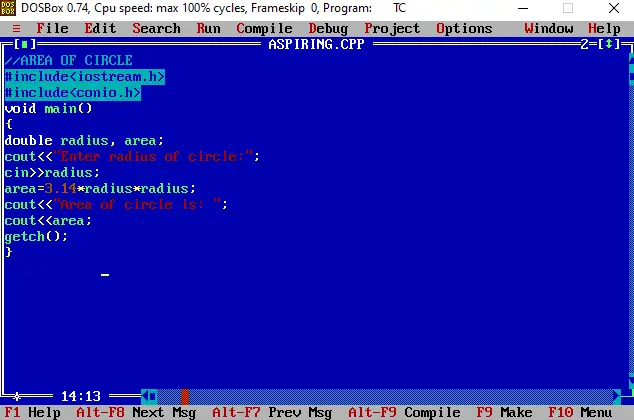
Working:
Flow of a program:
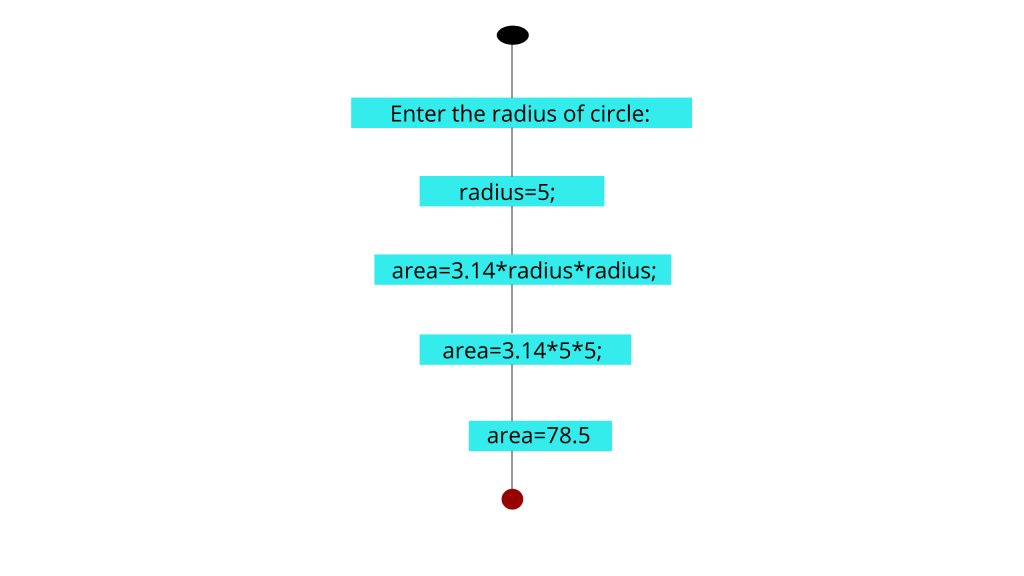
Find Out Area of Circle Using Function
Functions are used to perform certain actions, and they are important for reusing code: Define the code once and use it many times.
And, this is a another method to find out the area of circle at different sides i.e. by function.
Note: Always remember that execution starts from the main body only.
Example:
#include<iostream.h>
#include<conio.h>
double area_of_circle(int r)
{
double area=3.14*r*r;
cout<<"Area of a circle for radius "<<r<<"is: ";
return area;
void main()
{
cout<<area_of_circle(14)<<endl;
cout<<area_of_circle(5);
getch();
}
//Output:
Area of circle for radius 14 is: 615.44
Area of circle for radius 5 is: 78.5
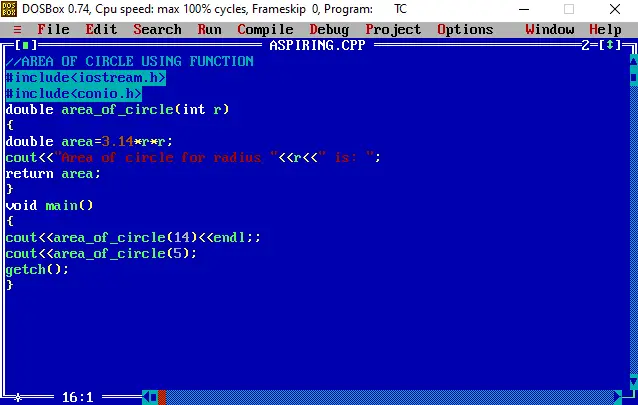
Working:
Flow of a program:
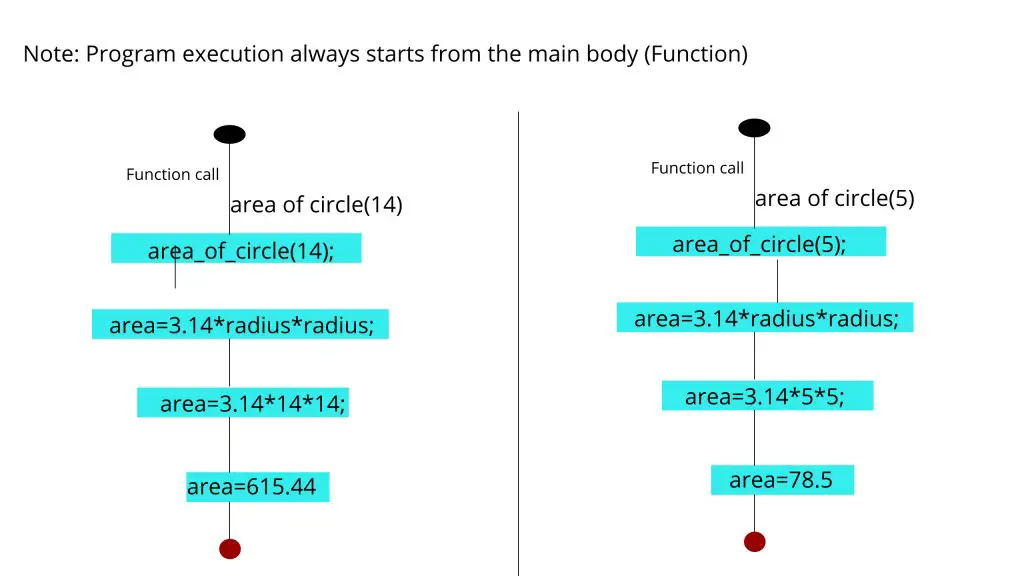
Well, I hope it really helped you to know C++ better.
Attention reader⚠ Don’t stop learning now.
Want to do more related programs? Just visit Area of Square.
Just stay with us to get more such exciting codes to explore more in C++.
View related posts:
- C++ Program to Add Two Matrices (Multi-dimensional Array)
- C++ Program to Add Two Numbers
- C++ Program to Calculate the Simple Interest
- C++ Program to Check Armstrong Number
- C++ Program to Check Palindrome Number
- C++ Program to Check Whether a Character is Vowel or Consonant
- C++ Program to Demonstrate the Arithmetic Operators
- C++ Program to Demonstrate the Assignment Operator
- C++ Program to Display Multi-Dimensional Array
- C++ Program to Display Single Dimensional Array
- C++ Program to Find Factorial of a Number
- C++ Program to Find Out Product of Digits
- C++ Program to Find Out Sum of Digits
- C++ Program to Find Out the Area of Square
- C++ Program to Find the Fibonacci Sequence
- C++ Program to Find the Largest Among Three Numbers
- C++ Program to Reverse a Number
- C++ Program to Swap Two Numbers
- C++ Program to Transpose the Matrix (2-D Array)